An array helper method is a built in function in programming languages like JavaScript that is specifically designed to work with arrays. These methods provide convenient and efficient ways to perform common operations on Arrays such as iteration over array elements, filtering elements, transforming elements, searching for elements.
Array helper methods are valuable because they simplify array manipulation tasks and make the code more readable and concise. Instead of writing complex loops and conditions to work with arrays, array helper methods can be used to achieve the same results with fewer lines of code.
foreach
foreach method intends to execute a provided function once for each array element. This method allows to iterate over each element of an array and apply a callback function to each element. The syntax is given as:
- ‘array’ : The array you want to iterate over.
- ‘callback’: A function to execute on each element of the array.
- ‘element’: The current element being processed in the array
- ‘index’ (optional): The index of the current element being processes.
- ‘array’ (optional): The array that ‘forEach’ is being applied to.
- ‘thisArg’ (optional): An object that will be passed as the ‘this’ value to the callback function. If not provided, ‘undefined’ will be used as the ‘this’ value.
The forEach method does not return anything. It iterates through the array and apploes the callback function to each element. The primary purpose of ‘forEach’ is to eecute some code for each item in the array without creating a new array or modifying the original one.
const numbers = [10, 20, 30, 40, 50];
numbers.forEach(function (num, id) {
console.log("num:", num);
});
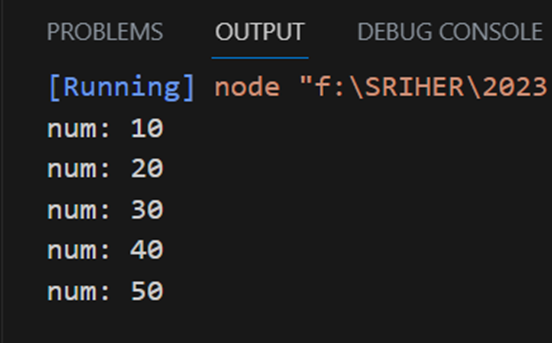
For each to process element in array of object
One can access the properties of the objects as well within the callback function. For example: to calculate and log the average for students who got greater than 45.
const student = [
{ name: "ABC", age: 18, m1: 89, m2: 93 },
{ name: "DEF", age: 20, m1: 100, m2: 99 },
{ name: "GFD", age: 21, m1: 82, m2: 44 },
];
let count = 0;
//Display all objects in array
student.forEach(function (stud, id) {
console.log("Student", id);
console.log(stud.name);
console.log(stud.age);
console.log(stud.m1);
console.log(stud.m2);
});
//Calculate average for students and display those students who got pass
student.forEach(function (stud, id) {
if (stud.m1 > 45 && stud.m2 > 45) {
let tot = stud.m1 + stud.m2;
let avg = tot / 2;
console.log("student ", id + 1);
console.log("Name=", stud.name);
console.log("Age=", stud.age);
console.log("M1", stud.m1);
console.log("M2", stud.m2);
console.log("Average", avg);
count++;
}
});
// finally print the number of students who got pass
console.log("Total Number of students who got pass", count);
Arrow function as callback function in forEach
One can access the properties of the objects as well within the callback function. For example: to calculate and log the average for students who got greater than 45.
const student = [
{ name: "ABC", age: 18, m1: 89, m2: 93 },
{ name: "DEF", age: 20, m1: 100, m2: 99 },
{ name: "GFD", age: 21, m1: 82, m2: 44 },
];
let count = 0;
//Calculate average for students and display those students who got pass
student.forEach((stud, id) => {
if (stud.m1 > 45 && stud.m2 > 45) {
let tot = stud.m1 + stud.m2;
let avg = tot / 2;
console.log("student ", id + 1);
console.log("Name=", stud.name);
console.log("Age=", stud.age);
console.log("M1", stud.m1);
console.log("M2", stud.m2);
console.log("Average", avg);
count++;
}
});
// finally print the number of students who got pass
console.log("Total Number of students who got pass", count);
map
The map() method is an array helper method that creates a new array by calling a provided function on each element of the original array. It returns a new array with the result of the function calls. The syntax is given as:
- ‘array’ : The original array you want to iterate over.
- ‘callback’: A function to execute on each element of the array.
- ‘element’: The current element being processed in the array
- ‘index’ (optional): The index of the current element being processes.
- ‘array’ (optional): The array that ‘mapi s being applied to.
- ‘thisArg’ (optional): An object that will be passed as the ‘this’ value to the callback function. If not provided, ‘undefined’ will be used as the ‘this’ value.
Example:
Create an array of cube of numbers
const numbers = [10, 20, 30, 40, 50];
const cubeNumbers = numbers.map(function (number) {
return number * number;
});
console.log(cubeNumbers);
Output
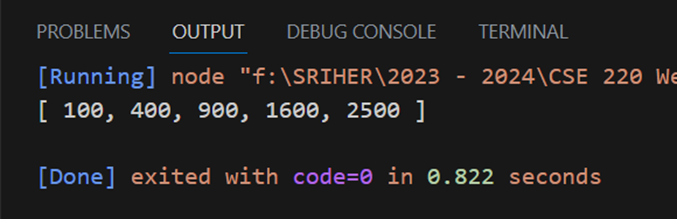
map( ) is used to create new array ‘names’ that contains only the names of the people from the ‘people’ array of objects.
const people = [
{ name: "John", age: 30 },
{ name: "Jane", age: 25 },
{ name: "Bob", age: 35 },
];
const names = people.map(function (person) {
return person.name;
});
console.log(names);
Output
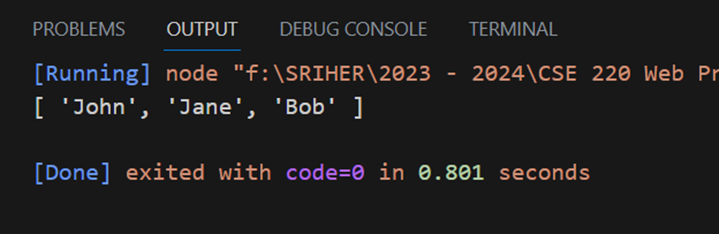
map() is a powerful method of transforming data in arrays and creating new arrays based on the values of the original array. It serves as an alternative to ‘forEach( )’ when there is a need to create a new array with modified values from the original one.
const celciustemperature = [10, 20, 30, 40, 50];
const FahrenheitTemp = celciustemperature.map((temp) => (temp * 9) / 5 + 32);
console.log(FahrenheitTemp);
Output
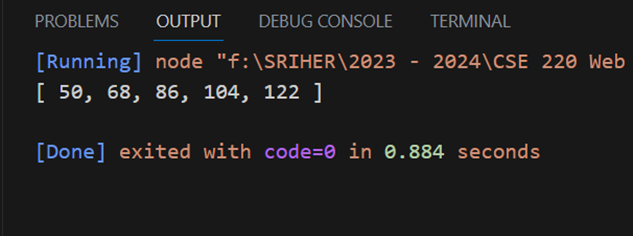
Implicit return: If an arrow function has only one expression, omit the curly braces ‘{}’ and the ‘return’ keyword. The result of the expression will be implicitly returned.
filter
It is an array helper method that creates a new array containing all the elements of the original array that pass a specific test ( provided as a callback function). It filters out elements that do not meet the criteria defined in the callback function. The syntax is given as:
- ‘array’ : The original array you want to filter
- ‘callback’: A function that will be called for each element in the array
- ‘element’: The current element being processes in the array
- ‘index’ (optional): The index of the current element being processed
- ‘array’ (optional); The array that ‘filter’ is being applied to
- ‘thisArg (Optional): An object to which ‘this’ will be set inside the callback function
Examples
Filter even numbers in an array
const numbers = [10, 11, 13, 14, 20, 22];
const evenNumbers = numbers.filter(function (num) {
return num % 2 == 0;
});
console.log(evenNumbers);
Output
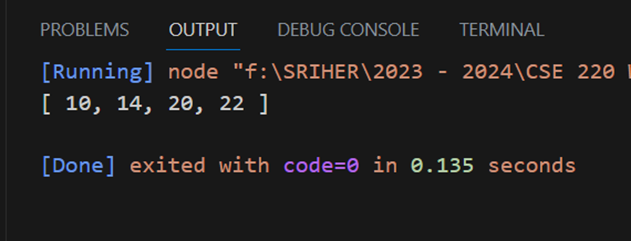
Find the products less than 400
const products = [
{ name: "Laptop", price: 800 },
{ name: "Smartphone", price: 500 },
{ name: "Tablet", price: 350 },
{ name: "Headphones", price: 100 },
];
// Find the products which are less than 400
const Newprod = products.filter(function (pname) {
return pname.price < 400;
});
console.log(Newprod);
/**
* Newprod.forEach(function (np) {
console.log("Name:", np.name, "\t", "Price:", np.price);
});
*/
Output
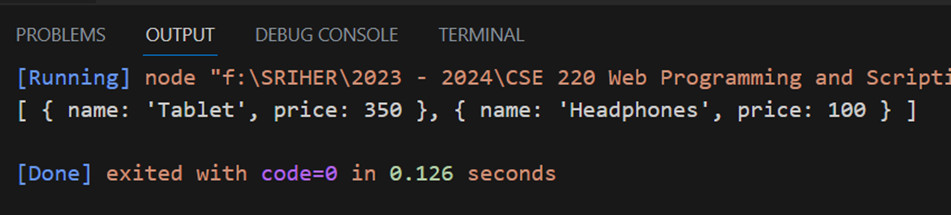
Filter method with arrow function
An array of objects named ‘ students’, where each object represents a student and contains their ‘name’, ‘age’ and ‘grade’.
Using the ‘filter( )’ method along with an arrow function, a new array called ‘students1’ is created that contains only the students with a grade of ‘A’.
const students = [
{ name: "John", age: 20, grade: "A" },
{ name: "Alice", age: 22, grade: "B" },
{ name: "abc", age: 18, grade: "C" },
{ name: "def", age: 19, grade: "A" },
];
// Find the students who got 'A'
const students1 = students.filter((stud) => stud.grade == "A");
console.log(students1);
Output
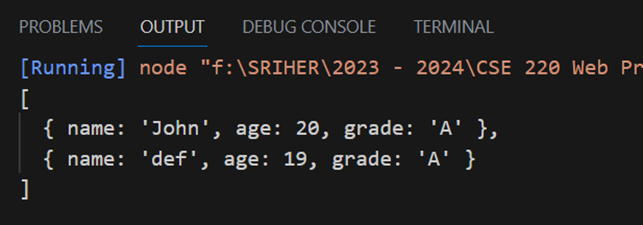
reduce
The reduce( ) method reduces the elements of an array to a single value. It executes a provided callback function for each element of the array (from left to right) and accumulates the results into a single value. The syntax is given as:
- ‘array’: The original array that you want to reduce
- ‘callback’: A function that will be created for each element in the array
- ‘collector’: This parameter stores the accumulated value after each iteration. It starts with the ‘initial value’ if provided, otherwise with the first element of the array. The value returned by the callback in each iteration becomes the new value of the collector.
- ‘current value’: This parameter represents the current element being processes in the array
reduce with function
- ‘index’: This parameter (optional) is the index of the ‘currentValue’ in the array.
- ‘array’: This parameter (optional) is the orignial array that ‘reduce’ is being applied to. It’s useful if you need to access the original array from within the callback.
- ‘initialValue’: This parameter(optional) is the initial value of the ‘collector’. It’s not required, but if provided, it will be starting value of the ‘collector’ in the first iteration
const student = [
{ name: "John", score: 85 },
{ name: "ABC", score: 82 },
{ name: "Bbb", score: 90 },
{ name: "ccc", score: 80 },
];
function TotalScore(collect, student) {
return collect + student.score;
}
const ts = student.reduce(TotalScore, 0);
console.log("Total Score:", ts);
Output
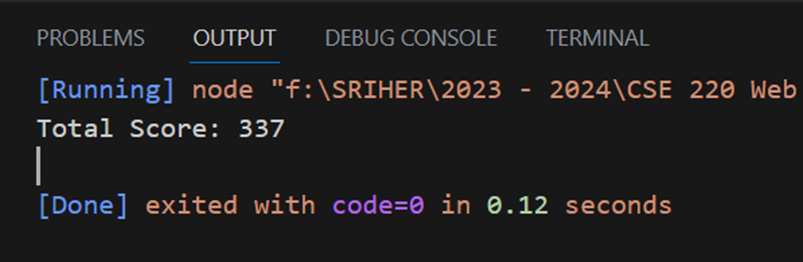
Example
Reduce with arrow
const students = [
{ name: "John", score: 85 },
{ name: "ABC", score: 82 },
{ name: "Bbb", score: 90 },
{ name: "ccc", score: 80 },
];
// Calculate the average score of all students
const totalScore = students.reduce(
(collect, student) => collect + student.score,
0
);
const averageScore = totalScore / students.length;
console.log("AverageScore = ", averageScore);
The arrow function (collect, student) => collect + student.score is used as a callback. Here, collect takes on the role of an intermediate variable that holds the cumulative collect of the scores as we iterate through each student.
After the reduce() operation, the collect variable contains the total score of all students.
Here’s how the process works:
Iteration 1: collect = 0, student.score = 85 collect after iteration: 0 + 85 Iteration 2: collect = 85, student.score = 82 collect after iteration: 167 Iteration 3: collect = 167, student.score = 90 collect after iteration: 257 Iteration 4: collect = 257, student.score = 80 collect after iteration: 337
Reduce with function expression
const students = [
{ name: "John", score: 85 },
{ name: "ABC", score: 82 },
{ name: "Bbb", score: 90 },
{ name: "ccc", score: 80 },
];
// Define a function expression for the callback
const TotalScore = function (collect, student) {
return collect + student.score;
};
// Calculate the average score of all students
const ts = students.reduce(TotalScore, 0);
const averageScore = ts / students.length;
console.log("Average Score =", averageScore);
Output
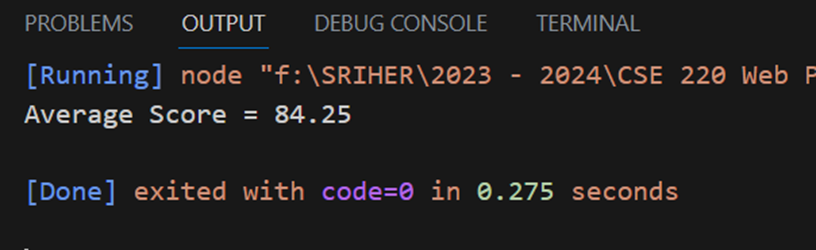
some
The function some() checks if atleast one element in the array passes the test implemented by the provided callback function. It returns a Boolean value ( true if atleast one element passes the test, otherwise false.
- ‘array’ : The array need to check
- ‘callback’: a function that will be called for each element in the array until the condition is satisfied
- ‘element’: The current element being processed in the array
- ‘index’ (optional): The index of the current element being processed
- ‘array’ (optional): The array that ‘some’ is being applied to
- ‘thisArg’ (optional): An object to which ‘this’ will be set inside the callback function
Example
The some() method is particularly useful when you need to determine if a certain condition is met by at least one element in the array. It stops iterating through the array as soon as it finds the first element that satisfies the condition, which can provide a performance advantage for large arrays.
const numbers = [10, 25, 7, 14, 30];
const res = numbers.some((number) => number > 20);
console.log(res);
Output
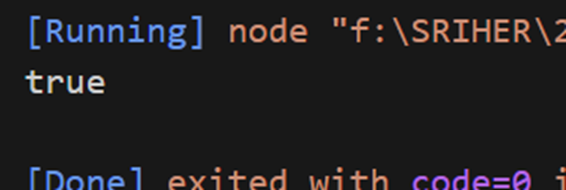
Example 2
const students = [
{ name: "John", score: 85 },
{ name: "ABC", score: 82 },
{ name: "Bbb", score: 89 },
{ name: "ccc", score: 80 },
];
const highScore = students.some((student) => student.score >= 90);
if (highScore) {
console.log("Atleast one student has mark greater than 90");
} else {
console.log("No student has a high score");
}
Output
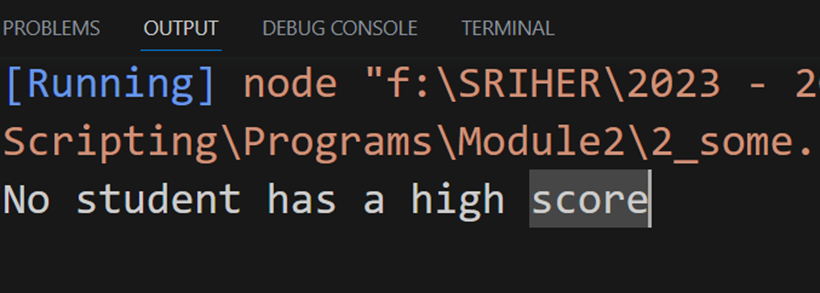
every
`every()` method checks if all the elements in the array pass the test implemented by the provided callback function. It returns a Boolean value (true if all elements pass the test, otherwise false)
- ‘array’ : The array to check
- ‘callback’ : A function that will be called for each element in the array until the condition is not satisfied
- ‘element’ : The current element being processed in the array
- ‘index’ (optional): The index of the current element being processed
- ‘array’ (optional): The arrat that ‘every is being applied to
- ‘thisArg’ (optional): An object to which ‘this’ will be set inside the callback function
Example
Create an array called students with 4 objects. The properties for each object are name and score which takes the value as specified below:
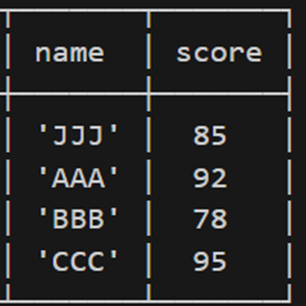
Check whether all the student score is greater than or equal to 70
const students = [
{ name: "JJJ", score: 85 },
{ name: "AAA", score: 92 },
{ name: "BBB", score: 78 },
{ name: "CCC", score: 95 },
];
const allPassing = students.every((student) => student.score >= 70);
if (allPassing) {
console.log("All students have passing scores.");
} else {
console.log("Not all students have passing scores.");
}
Output
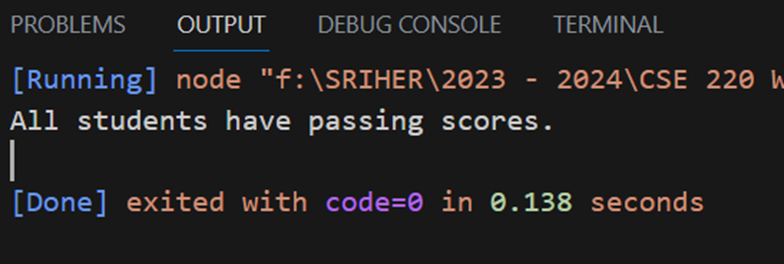
Check whether elements in the array are positive
var numbers = [2, 0, 12, 14, -2];
var result = numbers.every((num) => num > 0);
if (result) {
console.log("All numbers are positive");
} else {
console.log("Not All numbers are positive");
}
Output
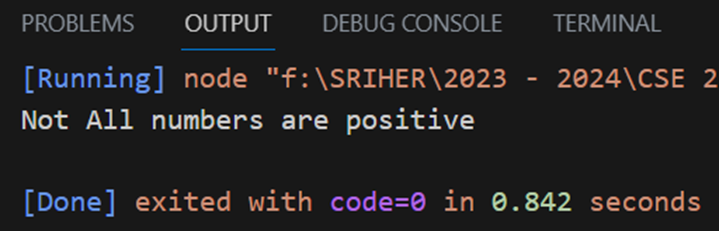
every() method is used to check if all elements in the array satisfy the specified condition. The callback function returns true if the condition is satisfied for all elements, and false if at least one element doesn’t meet the condition.
find
The ‘find()’ method is used to search for an element in an array based on a specified condition. It returns the first element in the array that satisfies the given condition. If no element satisfies the condition, ‘undefined’ is returned. The syntax is given as:
- ‘array’ : The array to search
- ‘callback’: A function that will be called for each element in the array until a matching element is found
- ‘element’ : The current element being processed in the array
- ‘index’ (optional): The index of the current element being processed
- ‘array’ (optional)” The array that ‘find’ is being applied to
- ‘thisArg’(optional) : An object to which ‘this’ will be set inside the callback function.
Example
Find the first even number
const arr = [3, 4, 1, 5, 9];
const res = arr.find((n) => n % 2 == 0);
if (res) {
console.log("First Even number:", res);
} else {
console.log("no number found:", res);
}
Output
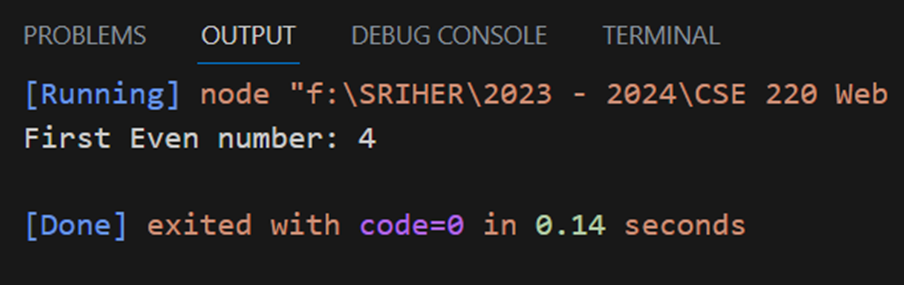
The find() method is used to search for an element in an array based on a provided condition. It returns the first element that satisfies the condition. If no element satisfies the condition, it returns undefined.
const fruits = ["apple", "banana", "cherry", "kiwi"];
const result = fruits.find((fruit) => fruit === "cherry");
if (result) {
console.log("Found:", result);
} else {
console.log("Not found");
}
Output
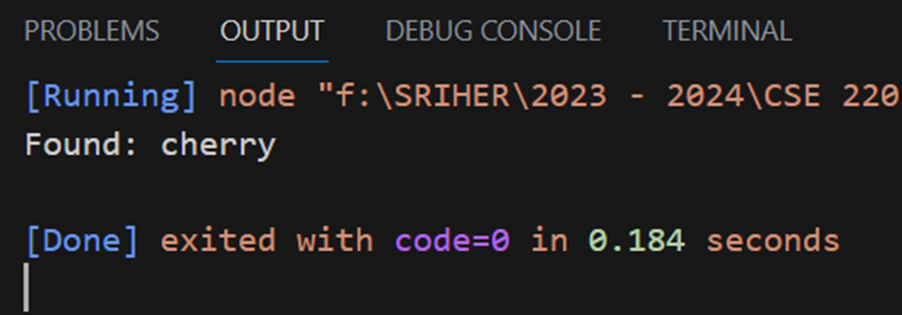
The find() method is used to find the first occurrence of the string “cherry” in the fruits array. The callback function checks if the current element is equal to “cherry”, and when it finds the first element that matches, it returns that element. If no match is found, it returns undefined.
Keep in mind that find() stops searching as soon as it finds the first matching element. If you want to find multiple elements that match the condition, you can use the filter() method instead.
Create an array called students with 4 objects. The properties for each object are name and score which takes the value as specified below:
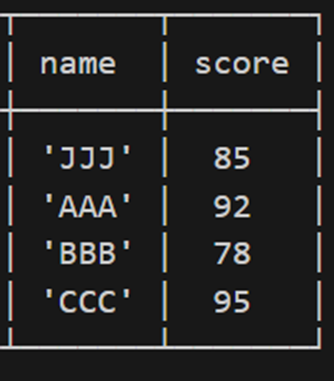
Find the student with highest score
const students = [
{ name: "JJJ", score: 85 },
{ name: "AAA", score: 92 },
{ name: "BBB", score: 78 },
{ name: "CCC", score: 95 },
];
const highScorer = students.find((student) => student.score >= 90);
if (highScorer) {
console.log("High scorer:", highScorer.name);
} else {
console.log("No high scorer found.");
}
Output
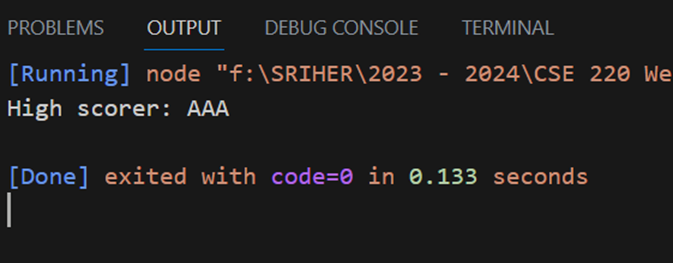
indexOf
‘indexOf()’ method can be used to find the index of the first occurrence of a specified element in an array. IF the element is not found in the array, it returns -1. The syntax is:
- ‘array’: The array to search in
- ‘searchElement’: The element to find in the array
- ‘fromIndex’ (optional): The index at which to start searching. If not specified, the search starts from the beginning of the array.
indexOf() method doesn’t work with arrow functions for comparison because it relies on reference equality.
indexOf() only finds the index of the first occurrence. If you need to find the index of subsequent occurrences, you may need to use indexOf() with a custom fromIndex, or consider using other methods like findIndex() for more complex scenarios.
Example
const fruits = ["apple", "banana", "cherry", "apple", "kiwi"];
const index1 = fruits.indexOf("apple");
const index2 = fruits.indexOf("cherry");
const index3 = fruits.indexOf("pear");
console.log(`Index of apple : ${index1}`);
console.log(`Index of cherry : ${index2}`);
console.log(`Index of pear : ${index3}`);
Output
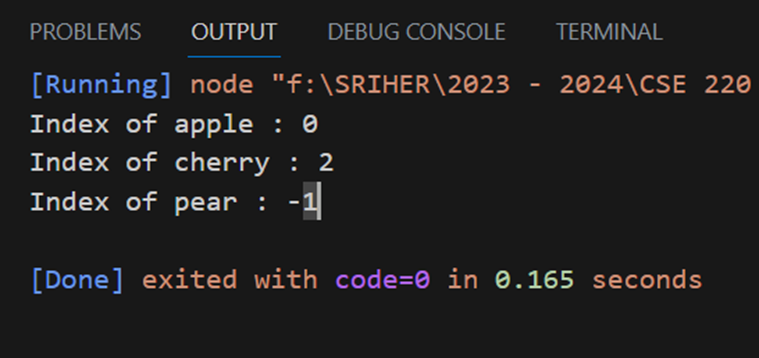
findIndex
The findIndex() method is a built in JavaScript array method that searches for an element in an array based on a provided condition and returns the index of the first element that satisfies the condition.
- ‘array’: The array one needs to search
- ‘callback’: A function that will be called for each element in the array until a matching element is found
- ‘element’: The current element being processed in the array
- ‘index’ (optional): The index of the current element being processed.
- ‘array’ (optional): The array that ‘findIndex’ is being applied to
- ‘thisArg’ (optional): An object to which ‘this’ will be set inside the callback function.
Example
Find the index of first number which is greater than 4.
const numbers = [2, 5, 8, 1, 4];
const index = numbers.findIndex((number) => number > 4);
if (index !== -1) {
console.log(`First number greater than 4 found at index ${index}`);
} else {
console.log("No number greater than 4 found.");
}
Output
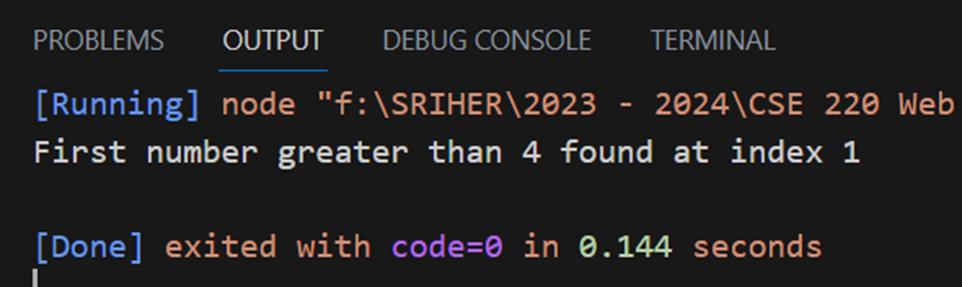
Find the index of the first occurrence of student whose name is ABC and score is 82
const students = [
{ name: "John", score: 85 },
{ name: "ABC", score: 82 },
{ name: "Bbb", score: 89 },
{ name: "ABC", score: 82 },
];
const searchStud = { name: "ABC", score: 82 };
const index1 = students.findIndex(
(student) =>
student.name === searchStud.name && student.score === searchStud.score
);
if (index1 != -1) {
console.log(`Found at index ${index1}`);
} else {
console.log(`Not found`);
}
Ouput:
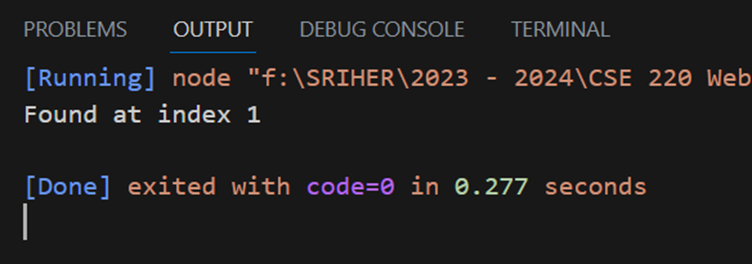
findIndex() method uses an arrow function as its argument. The arrow function compares both the name and score properties of each student object in the array with the searchStud object’s properties.
lastIndexOf
The ‘lastIndexOf() method is used to find the index of the last occurrence of a specified element in an array. If the element is not found in the array, it returns -1.
- ‘array’ : The array to search in
- ‘searchElement’ : The element to find in the array
- ‘fromIndex’ (optional): The index at which to start searching from the end of the array. If not specified, the search starts from the last element.
Example
To find the last occurrence
const fruits = ["apple", "banana", "cherry", "apple", "kiwi"];
const index1 = fruits.lastIndexOf("apple");
const index2 = fruits.lastIndexOf("cherry");
const index3 = fruits.lastIndexOf("pear");
console.log(`Index of apple : ${index1}`);
console.log(`Index of cherry : ${index2}`);
console.log(`Index of pear : ${index3}`);
Output
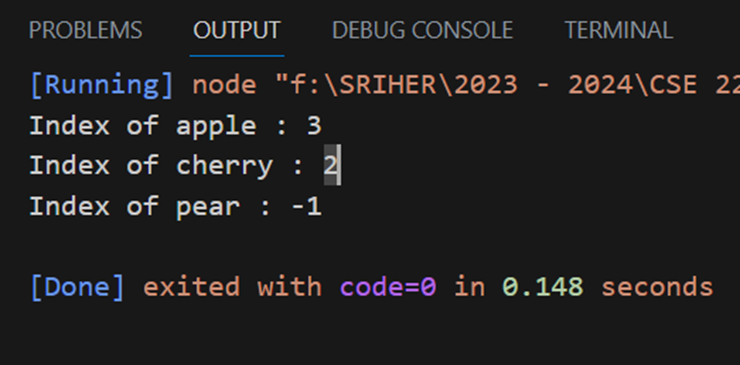
Views: 6