Variables life cycle
All the variables undergo three stages viz: Declaration, Initialization and Assignment.
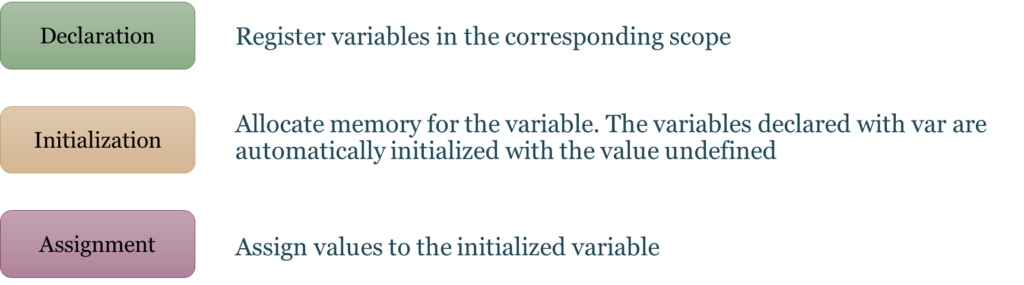
Declaration & Initialization
This is the first stage of a variable’s life cycle. Variables in JavaScript can be declared using the ‘var’, ‘let’, or ‘const’ keywords. The declaration process allocates memory for the variable and associates a name with it.
var age; // Declaring the variable age
let name; // Declaring the variable name
const PI = 3.14; // Declaration of constant variable
Variables can be initialized during declaration or at later stage. If a variable is declared with an initial value, it enters the initialization stage and memory is allocated to store the value assigned to it
var age = 32; // Initializing the variable age with the value 32
let name = “Raji” ; // Initializing the variable name with the value Raji
Assignment
Variables can have their values changed or updated after initialization and this process is known as assignment.
var age = 32; // Initialization of the variable ‘age’ with the value 32
age = 34; // Assignment, changing the value of age to 34
function myfun() {
console.log(a);
}
myfun();
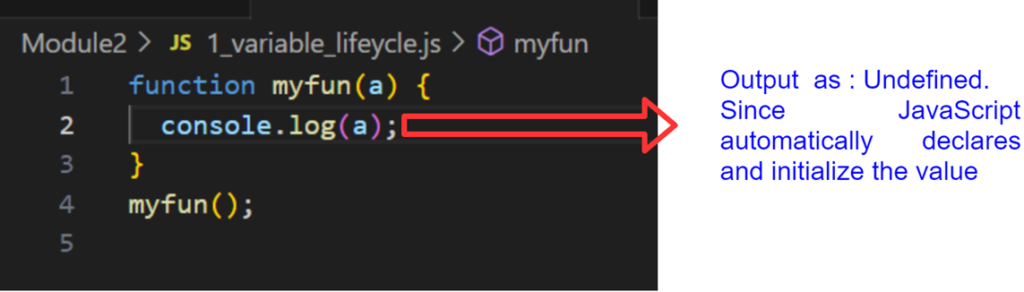
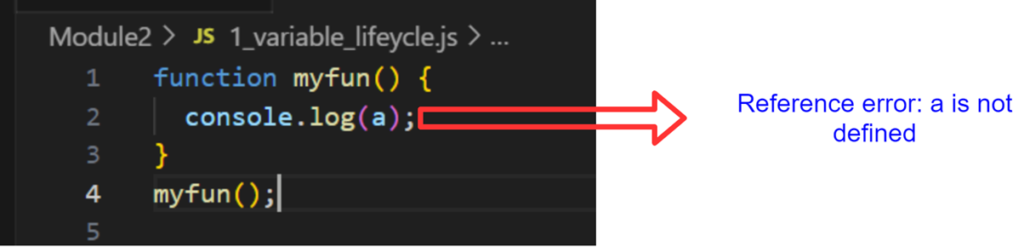
Lifecycle of var
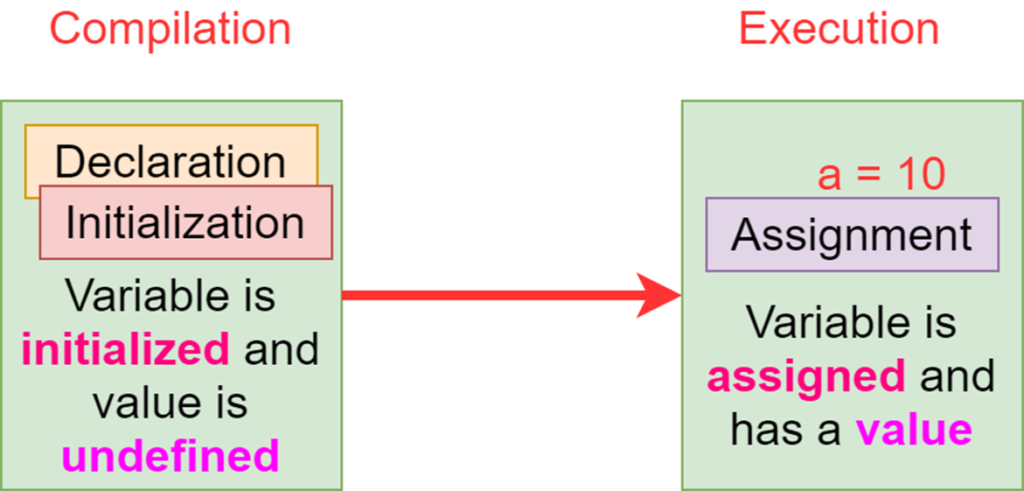
Declaration and Function Scope
Variables declared with ‘var’ are function – scoped, meaning they are accessible within the function where they are declared. If a variable is declared outside any function, it becomes a global variable, accessible throughout the entire program
function myfun() {
var x = 10; // Declaration of 'x' within this function
console.log(x); // output: 10
}
myfun();
//console.log(x);
Output
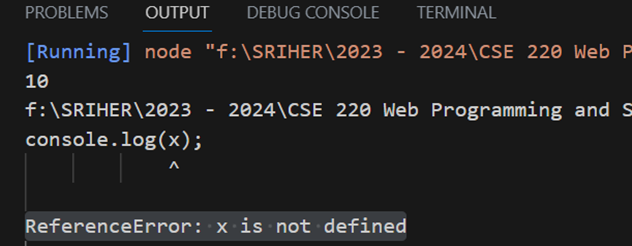
Hoisting
Variables declared with ‘var’ are hoisted to the top of their function or global scope during the creation phase of the execution context. This means that once can use the variable before its actual declaration in the code, but it will have the value ‘undefined until the declaration is reached.
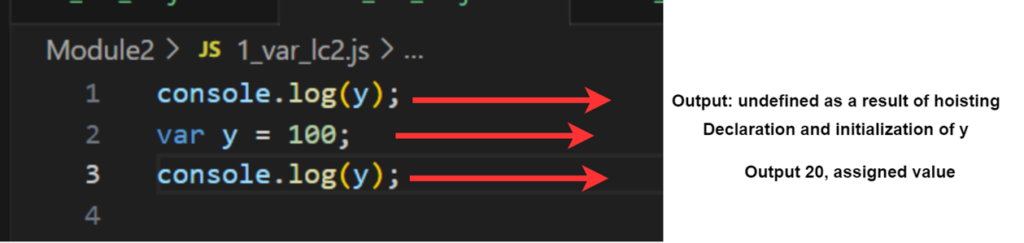
Output
console.log(y);
var y = 100;
console.log(y);
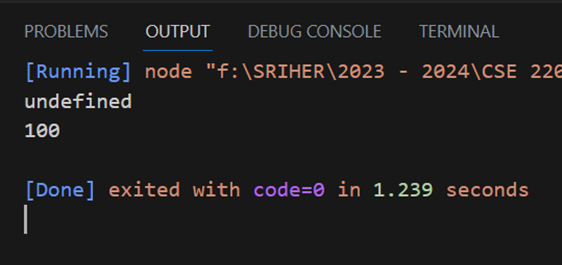
Initialization
‘var’ variables are automatically initialized with the value ‘undefined; during the hoisting phase
Redefinition and Reassignment
Variables declared with ‘var’ can be redeclared within the same scope without any errors, and they can also be reassigned new values.
var age = 30; // Declaration and initialization of 'age'
var age = 35; // Redefinition of 'age' (no error)
function myfun() {
var name = "ABC";
name = "Raji"; // Reassignment of name within the same function
var name = "DEF"; // Redefinition of 'name' (no error)
console.log(name); // Output:"DEF" the current value
}
myfun();
Output
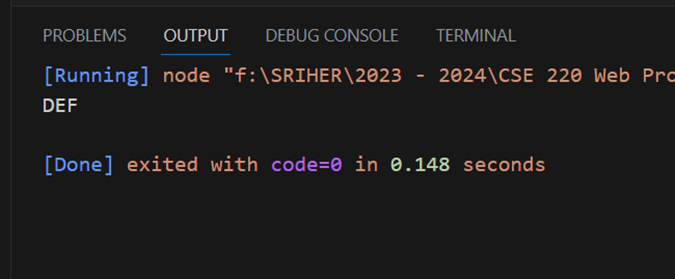
Lifecycle of let
Variables declared using the ‘let’ keyword have a lifecycle that involves various stages including block scope and temporal dead zone. The lifecycle of variables declared with ‘let’ can be summarized as follows
Declaration and Block Scope
When a variable is declared using ‘let;, it is block scoped meaning it is only accessible within the block or statement where it was declared, including any nested blocks.
{
let x = 10; // Declaration of 'x' within this block
console.log(x); // output: 10
}
console.log(x); // Error: 'x' is not defined,as it is outside the block
Temporal Dead Zone
Variables declared with ‘let; are subject to the Temporal Dead Zone, a period between the start of the block and the actual declaration of the variable. During this time, trying to access the variable results in a Reference Error
console.log(x); // Error cannot access ‘x’ before initialization
let x = 10; // Declaration of ‘x’ comes later in the code
Initialization
Unlike variables declared with ‘var’, ‘let’ variables are not automatically initialized with the value ‘undefined’. As a result, they remain in the temporal dead zone until their declaration is reached during execution.
let x; // Declaration without initialization
console.log(x); // Output: undefined
x = 20; // Initialization of 'x' with value 20
console.log("Now", x); // Output : 20
Reassignment
Variables declared with ‘let’ can have their values reassigned within their block scope.
let age = 20; // Declaration and initialization of 'age'
age = 24; // Reassignment, changing the value of 'age' to 35
console.log(age); // Output: 24, updated one
No redeclaration
Unlike variables declared with ‘var’, one cannot redeclare a variable with ‘let’ within the same scope. Attempting to do so will result in Syntax Error
let dept = "AIML"; // Declaration and Initialization of a variable
//let dept = "AIDA"; //SyntaxError: Identifier 'dept' has already been declared
Lifecycle of const
Variables declared using const keyword have a lifecycle that differs from both ‘var’ and ‘let’. The ‘const’ keyword is used to declare constants, which are variables that cannot be reassigned once they are initialized. The lifecycle of variables declared with ‘const’ can be summarized as:
Declaration and Block Scope
Like variables declared with ‘let’, variables declared with ‘const’ are block scoped, meaning they are accessible within the block or statement where they were declared, including any nested blocks.
{
const x = 10; // Declaration of 'x' within this block
console.log(x); // output: 10
}
console.log(x); // Error: 'x' is not defined,as it is outside the block
Temporal Dead Zone
Variables declared with ‘const’ are subject to Temporal Dead Zone, just like the variables declared with ‘let’. During this time, trying to access the variable before its declaration results in a Reference Error.
//const x; // SyntaxError: Missing initializer in const declaration
//console.log(x); // ReferenceError: Cannot access 'x' before initialization
const x = 20; // Initialization of 'x' with value 20
console.log("Now", x); // Output : 20
Initialization
Variables declared with ‘const’ must be initialized during the declaration. Once a value is assigned to a ‘const’ variable, it cannot be changed or reassigned.
const PI = 3.14; // Declaration and Initialization of PI
console.log(PI); // Output: 3.14
PI = 3.14159; // Error Assignment to constant variable
No Reassignment
The most significant difference between variables declared with ‘const’ and those declared with ‘let’ is that ‘const’ variables cannot be reassigned or have their values changed after initialization.
const age = 12; // Declaration and initialization of ‘age’
age = 24; // Error ; Assignment to constant variable
No Redeclaration
As with ‘let; one cannot redeclare a variable with ‘const’ within the same scope. Attempting to do so will result in a syntax error.
const age = 12; // Declaration and initialization of ‘age’
const age = 24; // Syntax Error: Identifier age has already been declared
Views: 16