Table of Contents
show
Example 8:
Create an object called ‘person’ with the following properties
- ‘name’ (string)
- ‘age’ (number)
- ‘gender’ (String)
- ‘isStudent’ (Boolean)
- Create a method called ‘greet’ for the ‘person’ object that logs a greeting message to the console, including the person’s name and age
- Access the ‘name’ property of the ‘person’ object and store it in a variable called ‘personName’
- Add a new property ‘city’ to the ‘person’ object and set its value to a city of your choice. Also, modify the ‘age’ property to increase it by 5
- Loop through the ‘person’ object and log each property and its value to the console
- Create another object called ‘address’ as a nested object within ‘person’ with the following properties,
- ‘street’ (string
- ‘postalCode’ (string)
- Access the ‘postalCode’ property inside the ‘address’ object and store it in a variable called ‘postC’
Code:
const person = {
name: "ABC",
age: 15,
gender: "Male",
isStudent: false,
greet: function () {
console.log(`Welcome,${this.name}, you are ${this.age} years old`);
},
};
//i) Access the ‘name’ property of the ‘person’ object
//and store it in a variable called ‘personName’
personName = person.name;
console.log("Name of the person : " + personName);
// ii) Add a new property ‘city’ to the ‘person’ object
//and set its value to a city of your choice.
person.city = "Chennai";
console.log("Now, city = ", person.city);
//Also, modify the ‘age’ property to increase it by 5
person.age = person.age + 5;
console.log("Now Age = ", person.age);
// iii) Loop through the ‘person’ object and
//log each property and its value to the console
console.log("Iterating the person object");
for (let i in person) {
console.log(person[i]);
}
//iv) Create another object called ‘address’ as a nested
//object within ‘person’ with the following properties
//‘street’ (string
// ‘postalCode’ (string)
const address = {
street: "123 Main Road",
postalCode: "62345",
};
person.address = address;
// v) Access the ‘postalCode’ property inside the ‘address’
//object and store it in a variable called ‘postC’
let postC = person.address.postalCode;
console.log("Post code : ", postC);
console.log("Printing property of person : ");
console.log(person);
person.greet();
Output:
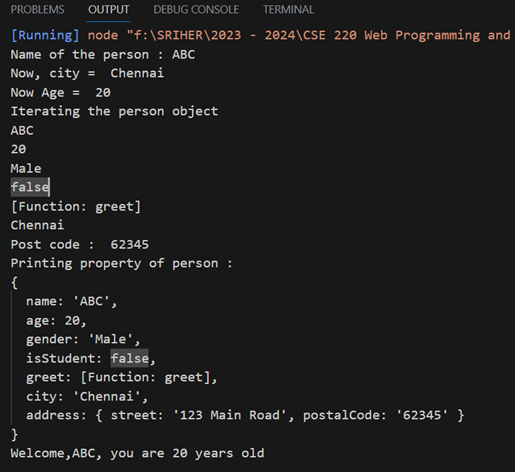
Views: 121