A template literal, also known as a template string, is a feature in JavaScript that allows to embed expressions and variables directly into strings. This provides a more concise and flexible way to create strings, especially when need to include dynamic content or multiline text
Template literals are enclosed by backticks (“) instead of single or double quotes, which are used for regular strings. Inside template literals, placeholders can be used to include values dynamically
When the template literal is evaluated, these placeholders are replaced by the actual values of the expressions. Some of the advantages are: String Interpolation, Multi-line String, Expression Evaluation, Escaping Special Characters and Tagged template literals
String Interpolation
Variables and expressions can be directly embedded within a string
const name = "ABC";
let mark = 90;
// using string concatenation
let cs = "My name is :" + name + " and my mark is : " + mark;
console.log(cs);
// using Template Literal - String Interpolation
let ts = `My name is : ${name} and my mark is : ${mark}`;
console.log(ts);
Output
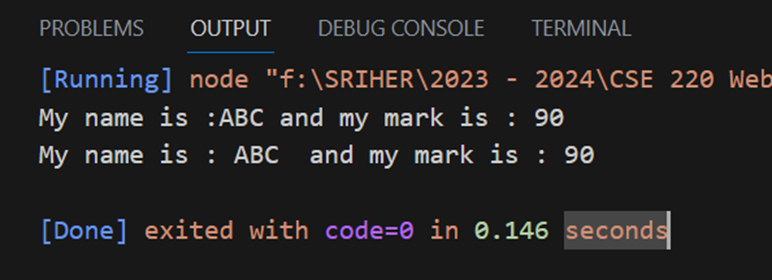
ØIn this example, we have a name variable and mark variable. We want to create a sentence that includes these values. Using string concatenation, we need to use the + operator to join the strings and variables.
ØWith template literals and string interpolation, we can directly include the variables within ${} placeholders in the string.
Multiline Strings
Template literals can span multiple lines without the need for concatenation or escaping line breaks.
const multilineString = `this is multi-
line string prepared using Template literal.
It does not use line break and
+ for concatenation.`;
console.log(multilineString);
console.log("\n");
const stringliteral =
"This is normal string. \n" +
"It requires line break \n and " +
" + for concatenation.";
console.log(stringliteral);
Output
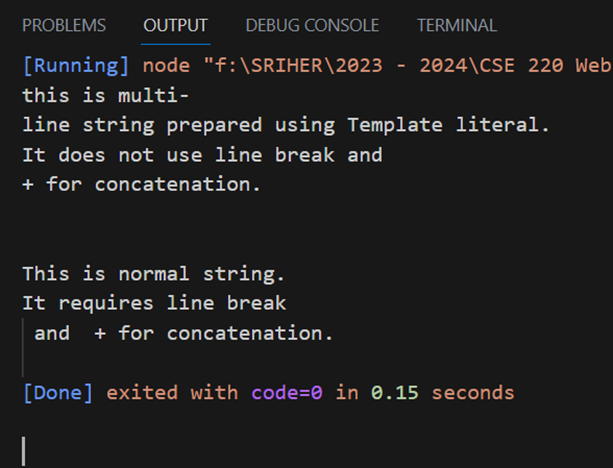
ØIn Template literal: the string content is enclosed within backticks (`), and it spans multiple lines naturally. The line breaks are preserved in the output without any additional effort.
ØIn String Literal: Each line of the string is separated by the + operator, and line breaks are indicated using the escape sequence \n. This approach is less readable and can become cumbersome when dealing with longer strings or more complex content.
ØTemplate literals are recommended for creating multiline strings, as they provide a more concise and readable way to achieve the same result
Expression Evaluation
Expressions inside `${}` are evaluated and replaced with their results
const x = 5;
const y = 10;
console.log(`sum of ${x} and ${y} is ${x + y}`);
Output
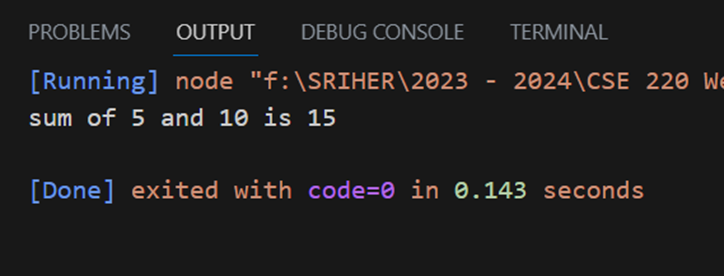
Escaping Special Characters
To escape characters inside template literals, use the backslash ‘\’ character before the character to escape.
Example
To escape backticks, dollar signs, backslashes, The escaped characters are treated as regular characters in the resulting string
const msg = `This is backticks \` and this is \$`;
const msg1 = `Line 1 \n Line 2 \n Indented line \t Line 3`;
console.log(msg);
console.log(msg1);
Output
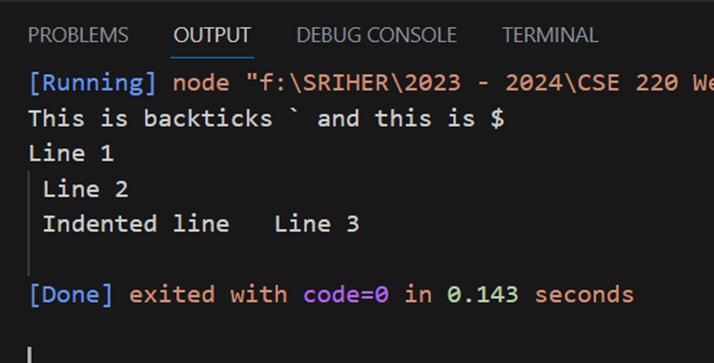
Tagged Template Literals
A tagged template is an advanced feature of template literals in JavaScript. It allows to customize the way template literals are processed by using a function, called a tag function, that is applied to the template literal’s parts and expressions. This enables to create powerful and flexible string manipulation functions. The syntax is given as
- `tagFunction` : A function that is applied to the template literal parts and expressions
- ‘template literal parts’: The parts of the template literal separated by expressions
- ‘expressions’: The values of expressions inside ‘${}’ placeholders
Example
Template literals can span multiple lines without the need for concatenation or escaping line breaks.
function emphasize(strings, ...values) {
console.log("Strings : ", strings); // Array of template literal parts
console.log("values : ", values); // Array of expression values
// console.log(values[0]);
}
const name = "abc";
const age = 30;
emphasize`Hello, my name is ${name} and I am ${age} years old.`;
function highlight(strings, ...values) {
console.log("Length of strings :", strings.length);
console.log("Length of values : ", values.length);
let result = "";
strings.forEach((string, index) => {
console.log("index: ", index);
result += string;
if (index < values.length) {
result += values[index].toString().toUpperCase();
}
});
return result;
}
const ht = highlight`Hello, my name is ${name} and I am ${age} years old.`;
console.log(ht);
Output
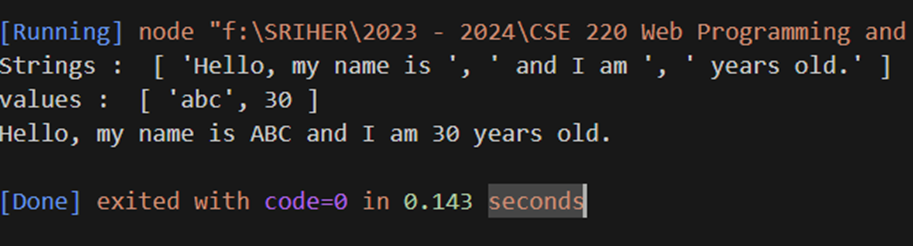
ØIn this part of the code, tag function called highlight is defined. This function processes the template literal and creates a modified string. It iterates over the strings array and concatenates the string part with the corresponding value from the values array. If there is a corresponding value, it converts it to uppercase using .toUpperCase(). Then, it returns the modified string.
ØThe emphasize function shows the raw breakdown of template parts and values, while the highlight function demonstrates a more advanced use case of processing and modifying the template literal’s content.
Views: 7