Looping Statements
For
The for loop is a control flow statement in JavaScript that allows to execute a block of code repeatedly for a specific number of times or based on a defined condition. The for loop consists of three parts within its parentheses, separated by semicolons:
Initialization: The loop variable is initialized before the loop starts. It usually sets the initial value of a counter variable
Condition: The loop continuous executing as long as the condition is true. It is evaluated before each iteration of the loop.
Increment / Update: The loop variable is updated after each iteration. It typically increments or decrements the counter variable.
The general structure of for loop is:
for(Initial Statement; Condition; Iteration Action)
{
//code
}
Example
Code
Loop to display iteration number from 0 to 5
for (let i = 1; i <= 5; i++) {
console.log("I am iteration:", i);
}
Output
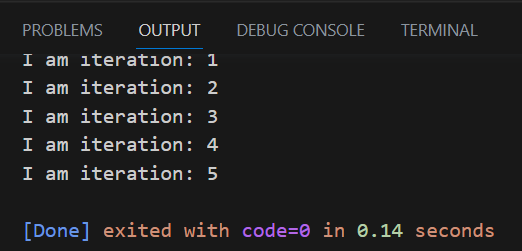
Loop to display from 5 to 1
Code
for (let i = 5; i >= 1; i--) {
console.log("I am iteration:", i);
}
Output
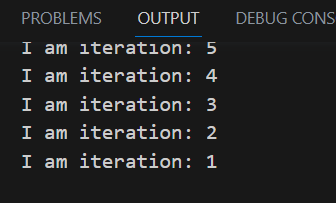
Loop to display the elements in array
Code
subjects = ["Tamil", "English", "Maths"];
for (let i = 0; i < subjects.length; i++) {
console.log("Subject:[", i, "]:" + subjects[i]);
}
Output
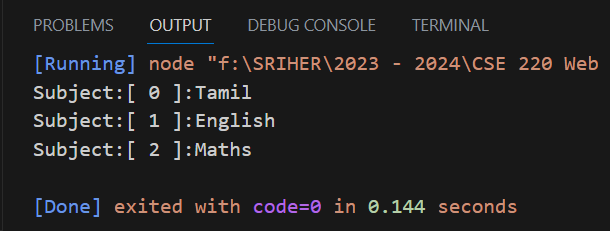
The for loop is used to iterate over the arrays and to execute the code for a specific number of times.
While
Another conditional control flow statement is while loop. It allows to execute the block of code repeatedly as long as specified condition is true. Unlike ‘for’ loop it does not have initialization or increment / update step. Rather it relies on condition to control the loop execution. The general syntax of while loop is as follows:
while(Condition)
{
//code
}
Working of while loop is as follows:
- The condition is evaluated before the loop starts. If the condition is true, the code inside the loop’s block is executed
- After executing the block of code, the condition is evaluated again. If it is still true, the block is executed again. The process continues until the condition becomes false.
- If the condition is false while checking the condition for first time, the code inside the loop’s block is never executed.
Example
Code: Sum of elements in array
let a = [20, 10, 30, 50, 40];
let i = 0;
let sum = 0;
while (i < a.length) {
sum = sum + a[i];
i++;
}
console.log("sum=", sum);
Output
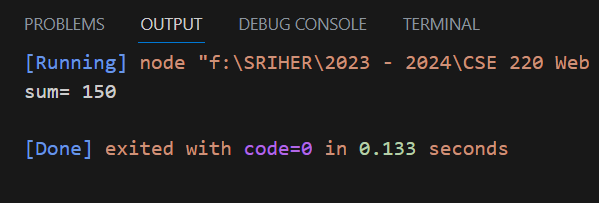
Code: Loop through an array and display its elements
let fruits = ["apple", "banana", "orange"];
let index = 0;
while (index < fruits.length) {
console.log(fruits[index]);
index++;
}
Output
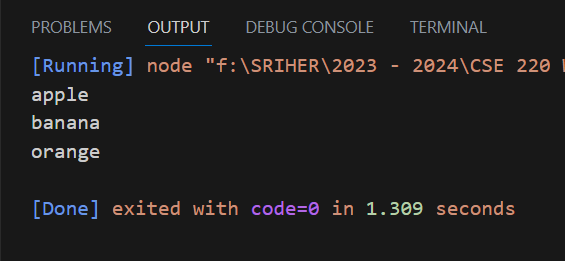
The while is useful when there is need to repeat code based on condition and don’t have a specific number of iterations in advance. It is necessary to ensure to avoid infinite loops, make sure the condition eventually becomes false to exit the loop
Do… While
The “do.. while” loop is a variation of the “while” loop. It allows to execute a block of code repeatedly as long as a specified condition is true. However, unlike the ‘while’ loop, the ‘do..while’ loop executes the code block at lease once before checking the condition. This means that the code inside the loop will always execute at least once regardless of whether the condition is true from the beginning. The syntax of do..while loop is as follows:
do
{
//code
}
while(condition)
The working of do..while loop is as:
- The code inside the body of the loop will be executed first regardless of whether the condition is true or false
- After executing the block of statements once, the condition is evaluated
- If the condition is true, then the block will be executed again until the condition becomes false.
Example
Code: Sum of numbers in an array
let a = [10, 20, 30];
let index = 0;
let sum = 0;
do {
sum = sum + a[index];
index++;
} while (index < a.length);
console.log("sum = ", sum);
Output
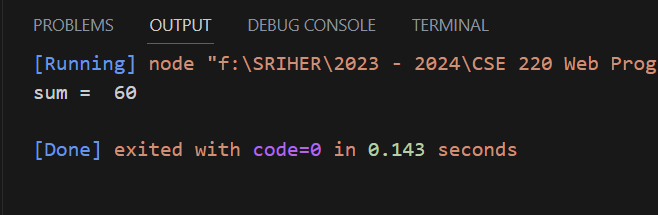
Code: Factorial of a number using do..while loop
const prompt = require("prompt-sync")();
let n;
n = prompt("Enter the number");
n = parseInt(n);
let fact = 1;
let i = 1;
do {
fact = fact * i; // fact *= i
i++;
} while (i <= n);
console.log("Factorial = ", fact);
Output
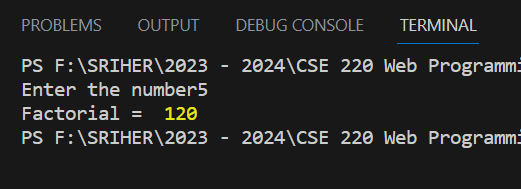
Conditional Statements
Conditional statements allow to execute different blocks of code based on specified conditions. They control the flow of the program, making decisions and taking actions based on whether certain conditions are true or false.
if
The ‘if’ statement allows to execute a block of code if a specified condition is true. If the condition is false, then the block of statement will not be executed. The syntax of if statement is given as:
if(condition)
{
//code
}
The condition is specified inside the parenthesis followed by the keyword if. The condition inside the parenthesis will be evaluated which evaluates to a Boolean value either True or False. If the condition is true, the code inside the curly braces will be executed. If the condition is false, then the block of statements is not executed and the control will move to the next statement after if block.
Code
const prompt = require("prompt-sync")();
let n;
n = prompt("Enter the number");
n = parseInt(n);
if (n > 10) {
console.log("The number is greater than 10.");
}
Output

This example, prompts the user to enter a number. If the entered number is less than 10, no action will be taken. If the entered number is greater than 10, then the message, ”The number is greater than 10” will be displayed.
if..else
The if…else statement is used to provide alternative code blocks to be executed based on a specific condition. If the condition on the ‘if’ statement is true, the code inside the ‘if’ block is executed. If the condition is false, the code inside the ‘else’ block is executed. The syntax of if..else is as follows:
if(condition)
{
//code block 1
}
else
{
//code block 2
}
- The condition is specified inside the parenthesis of if statement. It evaluates to Boolean value wither true or false
- If the condition is true, the code inside the curly braces {} will be executed and the ‘else’ block will be skipped
- If the condition is false, the code inside the ‘else; block will be executed and the ‘if’ block will be skipped
Example
Code
const prompt = require("prompt-sync")();
let age = prompt("Enter the age");
age = parseInt(age);
if (age >= 18) {
console.log("Eligible to vote");
} else {
console.log("Not Eligible to vote");
}
Output
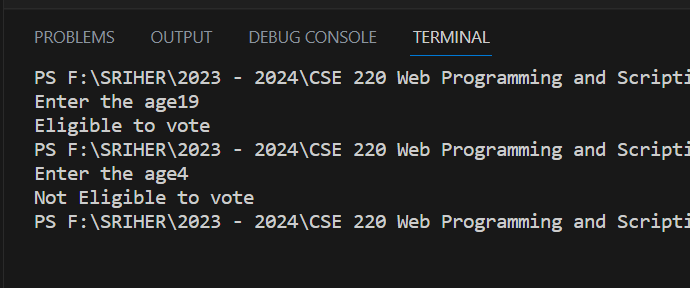
In this example, if the value of age is greater than or equal to 18, the code inside the if statement will be executed. If the value of age is less than 18, then the code inside the else part will get executed.
if.. else if…else
The if…else if.. else statement is used in JavaScript to conditionally execute different blocks of code based on multiple specified conditions. It allows to check multiple conditions and execute different code blocks accordingly. The syntax of if..elseif..else is given as:
if(condition 1)
{
//code block 1
}
else if(condition 2)
{
//code block 2
}
else
{
//code block 3
}
Example
Code
function Greetings() {
var today = new Date();
var hour = today.getHours();
var minu = today.getMinutes();
var sec = today.getSeconds();
if (hour < 12) {
console.log("GoodMorning !!! Time is" + hour + ":" + minu + ":" + sec);
} else if (hour > 12 && hour < 16) {
console.log("Good Noon !!!TIme is" + hour + ":" + minu + ":" + sec);
} else {
console.log("Good Day !!!TIme is" + hour + ":" + minu + ":" + sec);
}
}
Greetings();
Output
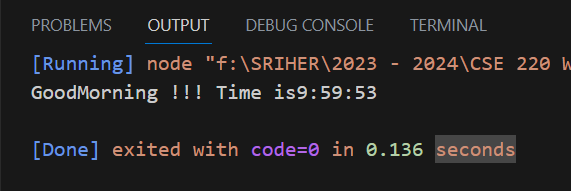
The above example, get the hour, minutes and seconds from date object. If the value is less than 12, it will print Good Morning with the current time. If the value is greater than 12 and less than 16, then it displays Good afternoon. Else, it will print Good Day.
switch… case..
The switch statement is another way to conditionally execute different blocks of code based on different cases or values of an expression. It is an alternative way of using if.. else if.. else statements when there is need to check a single expression against multiple possible values. The basic syntax of ‘switch’ statement is as follows:
switch(expression)
{
case 1:
//code
break;
case 2:
//code
break;
....
default:
//default block
break;
}
Example
const prompt = require("prompt-sync")();
let n = prompt("Enter a number");
n = parseInt(n);
switch (n) {
case -2:
console.log("The number is -2");
break;
case 0:
console.log("The number is 0");
break;
case 2:
console.log("The number is 2");
break;
default:
console.log("Some other number");
break;
}
Output
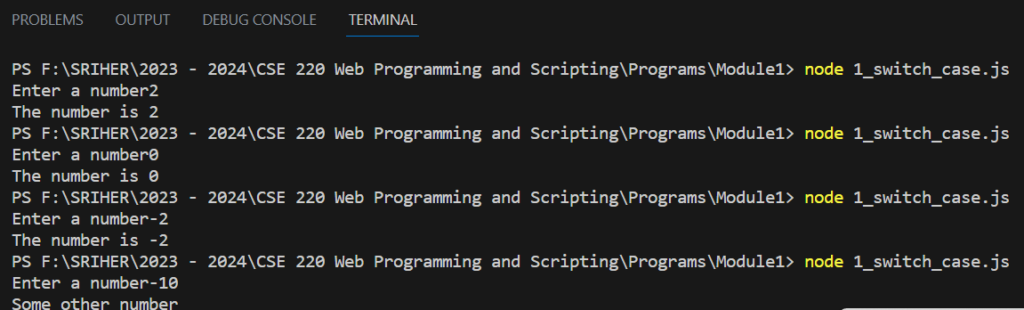
break
The break statement is used to terminate the execution of a loop or switch statement. It is commonly used inside loops or switch cases to exit the loop or switch block prematurely when a certain condition is met. The syntax is:
break;
In loops
When the break statement is encountered inside a loop, it immediately exits the loop and the program continues executing the code that follows the loop.
Example
Code
for (let i = 1; i < 10; i++) {
if (i == 3) {
console.log("I ll stop the loop");
break;
}
console.log(i);
}
Output
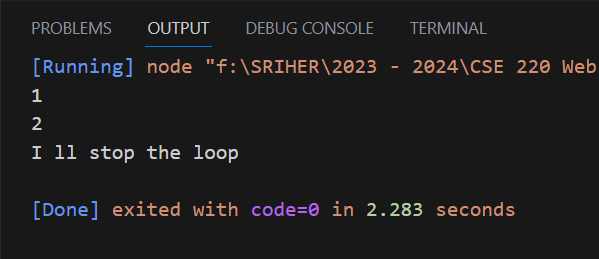
In switch case
When the break statement is encountered inside a ‘switch’ case, it exits the ‘switch’ block preventing the execution of subsequent cases.
const prompt = require("prompt-sync")();
let month = prompt("Enter the month");
month = parseInt(month);
let year = prompt("Enter the year");
year = parseInt(year);
let daycount;
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
daycount = 31;
console.log("Number of days=" + daycount);
break;
case 4:
case 6:
case 9:
case 11:
daycount = 30;
console.log("Number of days=" + daycount);
break;
case 2:
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
daycount = 29;
console.log("Number of days=" + daycount);
} else {
daycount = 28;
console.log("Number of days=" + daycount);
}
break;
default:
console.log("Invalid Month");
break;
}
Output

continue
The continue statement is used inside loops (such as ‘for’, ‘while’ or ‘do.. while’) to skip the current iteration and proceed to the next iteration of the loop. When the ‘continue’ statement is encountered, the remaining code inside the loop for the current iteration is skipped, and the loop proceeds with the next iteration, if any.
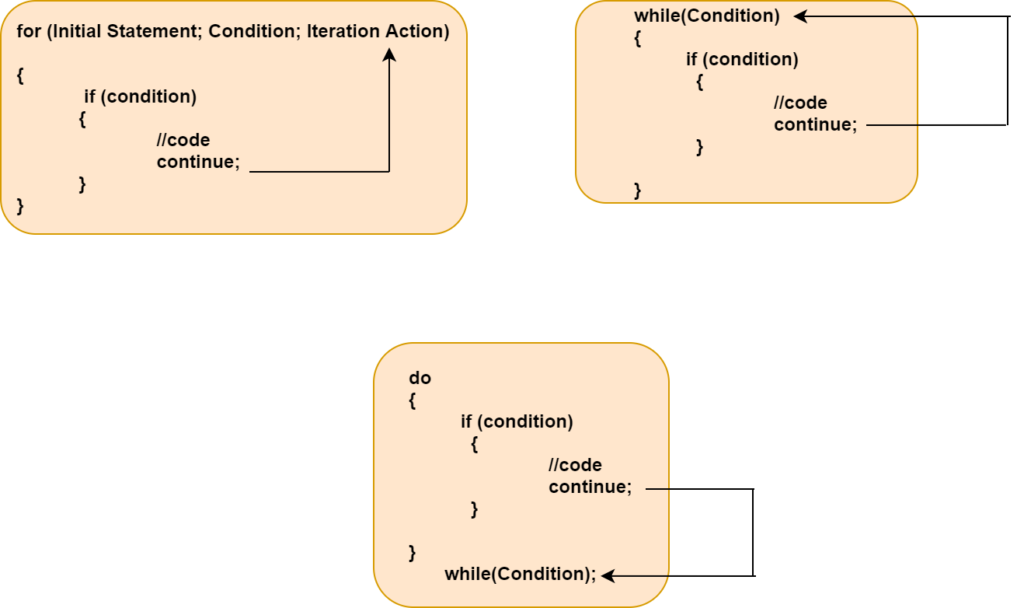
Example
Code
for (let i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue;
}
console.log(i);
}
Output
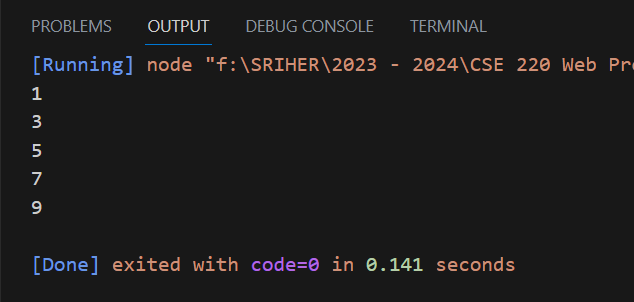
In this example, the if condition is true for all even numbers, thus continue statements take the control to the beginning of the loop making to print only odd numbers
Code
let i = 0;
while (i < 10) {
if (i % 3 == 0) {
//console.log(i);
i++;
continue;
}
console.log(i);
i++;
}
Output
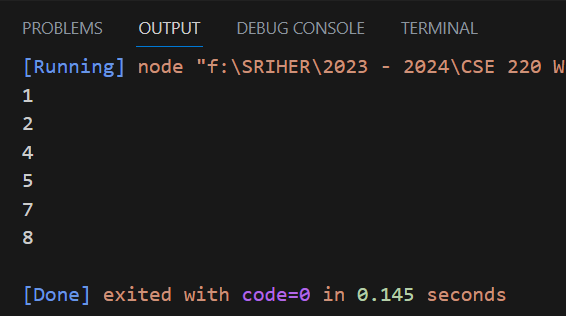
In this example, divisors of 3 are skipped by using continue statement inside if condition.
let i = 0;
do {
if (i % 2 == 0) {
i++;
continue;
}
console.log(i);
i++;
} while (i < 10);
In this example, the if condition is true for all even numbers, thus continue statements take the control to the beginning of the loop making to print only odd numbers
Views: 15