Table of Contents
show
Example: 5B
Illustrating array helper methods in array of objects
Consider the following Array of objects,
employees= [ {ID:123, empName:"kane", department:"HR", salary:65000, age:32},
{ID:100, empName:"Jane ", department: "office”, salary:45000, age:25},
{ID:1041, empName:"Liz ", department: "MBA “, salary:39000, age:45},
{ID:1008, empName:"Mcfe ", department: “Science ",salary:25000,age:29} ];
Develop a JavaScript code for,
- Display the employees who are getting more than 40000 Salary.
- Display the Employees details in alphabetical order.
- Find and display the total salary paid to all the employees of the organization.
- Find the maximum salary of the employees.
- Find the minimum salary of the employees.
- Find employees who are less than 30 years old.
Provide a bonus of Rs. 5000 for the employees who are getting less than 30000
Script
const employees = [
{ ID: 123, empName: "kane", department: "HR", salary: 65000, age: 32 },
{ ID: 100, empName: "Jane", department: "Office", salary: 45000, age: 32 },
{ ID: 123, empName: "Liz", department: "MBA", salary: 39000, age: 45 },
{ ID: 1008, empName: "McFe", department: "Science", salary: 25000, age: 29 },
];
// i. Find and Display employees earning more than 40000 salary
console.log("Employees earning more than 40000 salary:");
const highSalaryEmployees = employees.filter(
(employee) => employee.salary > 40000
);
highSalaryEmployees.forEach((employee) => {
console.log(
`ID: ${employee.ID}, Name: ${employee.empName}, Salary: ${employee.salary}`
);
});
// ii. Display the Employees details in alphabetical order
console.log("Employees details in alphabetical order (by name):");
const sortedEmployees = employees.sort((a, b) =>
a.empName.localeCompare(b.empName)
);
sortedEmployees.forEach((employee) => {
console.log(
`ID: ${employee.ID}, Name: ${employee.empName}, Department: ${employee.department}, Salary:
${employee.salary}`
);
});
// iii. Find and display the total salary paid to all employees
const totalSalary = employees.reduce(
(total, employee) => total + employee.salary,
0
);
console.log("Total salary paid to all employees:", totalSalary);
// iv. Find maximum salary
const maxSalary = employees.reduce(
(max, employee) => (employee.salary > max ? employee.salary : max),
employees[0].salary
);
console.log("Maximum Salary:", maxSalary);
// v. Find minimum salary
const minSalary = employees.reduce(
(min, employee) => (employee.salary < min ? employee.salary : min),
employees[0].salary
);
console.log("Minimum Salary:", minSalary);
// vi. Find employees less than 30 years old
const youngEmployees = employees.filter((employee) => employee.age < 30);
console.log("Employees less than 30 years old:");
youngEmployees.forEach((employee) => {
console.log(
`ID: ${employee.ID}, Name: ${employee.empName}, Age: ${employee.age}`
);
});
// vii) Give bonus to employees with salary less than 30000
const bonusEmployees = employees.map((employee) => {
if (employee.salary < 30000) {
return { ...employee, salary: employee.salary + 5000 };
}
return employee;
});
console.log("Employees with bonus:");
bonusEmployees.forEach((employee) => {
console.log(
`ID: ${employee.ID}, Name: ${employee.empName}, Salary: ${employee.salary}`
);
});
Output
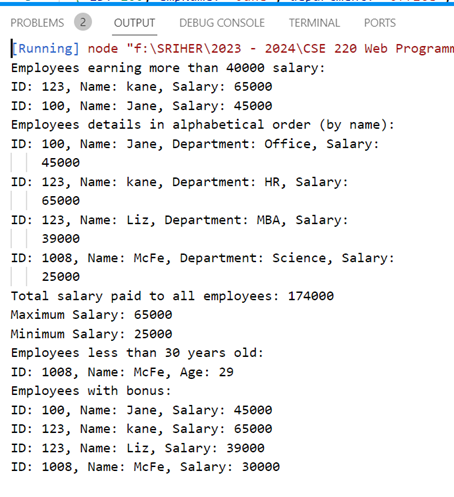
Views: 19