Example: 7
Illustration the concept of ES6 class, prototypes and function constructor
Explain the ES6 Class Approach. Describe how the `Employee` class is structured, including its constructor function and the `calculateBonus` method. Provide an example of creating an employee instance using this approach, specifying the properties like name, age, and salary, and calculate the bonus for the created employee instance.
Analyze the Prototype Approach. Explain how the `EmployeePrototype` function constructor is used, including the properties such as name, age, salary, and bonus. Describe the prototype-based method for calculating bonuses and provide an example of creating an employee instance using this approach, specifying the properties and calculating the bonus.
Explore the Function Constructor Approach. Describe the `createEmployee` function, including how it creates employee objects with properties like name, age, salary, and bonus. Explain the method for calculating bonuses in this approach. Create an employee instance using this approach, specifying the properties, and calculate the bonus. Additionally, demonstrate how the `greetFun` method is used for this employee instance.”
Script
// ES6 Class Approach
class Employee {
constructor(name, age, salary) {
this.name = name;
this.age = age;
this.salary = salary;
}
calculateBonus() {
return this.salary * 0.1; // Assuming 10% bonus
}
}
// Prototype Approach
function EmployeePrototype(name, age, salary, bonus) {
this.name = name;
this.age = age;
this.salary = salary;
this.bonus = bonus;
}
EmployeePrototype.prototype.calculateBonus = function () {
return this.salary * this.bonus;
};
// Function Constructor Approach
function createEmployee(name, age, salary, bonus) {
const employee = Object.create(employeePrototype);
employee.name = name;
employee.age = age;
employee.salary = salary;
employee.bonus = bonus;
return employee;
}
const employeePrototype = {
calculateBonus() {
return this.salary * 0.05; // Assuming 5% bonus
},
greetFun() {
console.log(`Hello from ${this.name}`);
},
};
// Creating instances using different approaches
const emp1 = new Employee("ABC", 30, 50000);
console.log(`Bonus for ${emp1.name}: $${emp1.calculateBonus()}`);
const emp2 = new EmployeePrototype("BBB", 25, 60000, 0.15);
console.log(`Bonus for ${emp2.name}: $${emp2.calculateBonus()}`);
const emp3 = createEmployee("CCC", 40, 75000, 0.08);
console.log(`Bonus for ${emp3.name}: $${emp3.calculateBonus()}`);
emp3.greetFun();
Output
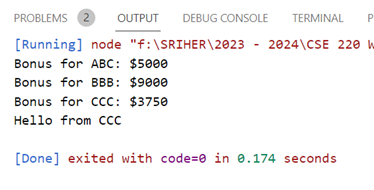
Views: 30