Table of Contents
show
The following conditional statements are available in python.
- Conditional if
- Alternative if .. else
- Chained if… elif…else
- Nested if.. else
Conditional if
Conditional (if) is used to test a condition, if the condition is true, the statements inside “if” will be executed.
Syntax
if(condition 1):
Statement
Flowchart
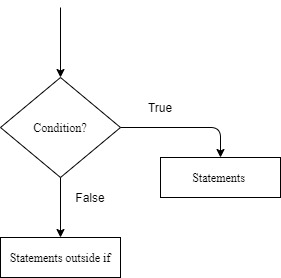
Example,
age = int(input('Enter the age'))
if(age >= 18):
print("Eligible to vote")
print("Illustration of Conditional If")
Output
Output 1:
Enter the age 21
Eligible to vote
Illustration of Conditional If
Output 2:
Enter the age 17
Illustration of Conditional If
Alternative if.. else
When a condition is true, the statement under if part is executed and when it is false the statement under else part is executed
Syntax,
if(condition):
Statement 1
else:
Statement 2
Flowchart
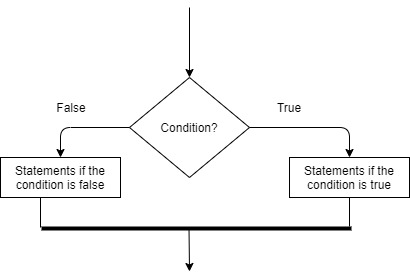
Example,
age = (int(input("Enter age")))
if(age >= 18):
print("You are eligible to vote")
else:
print("You are not eligible to vote")
Output
Output 1:
Enter age 21
You are eligible to vote
Output 2:
Enter age 15
You are not eligible to vote
Chained if .. elif… else
- elif is short for else if
- This is used to check more than one condition
- If the condition 1 is false, it checks for condition 2 of the elif block. If all the conditions are false, then the else part is executed
- Among the several if.. elif.. else part, only one part is executed according to the condition
- The if block can have only one else block. But it can have multiple elif blocks
Syntax,
if(condition 1):
Statement 1
elif(condition 2):
Statement 2
elif(condition 3):
Statement 3
else:
Default statement
Flowchart
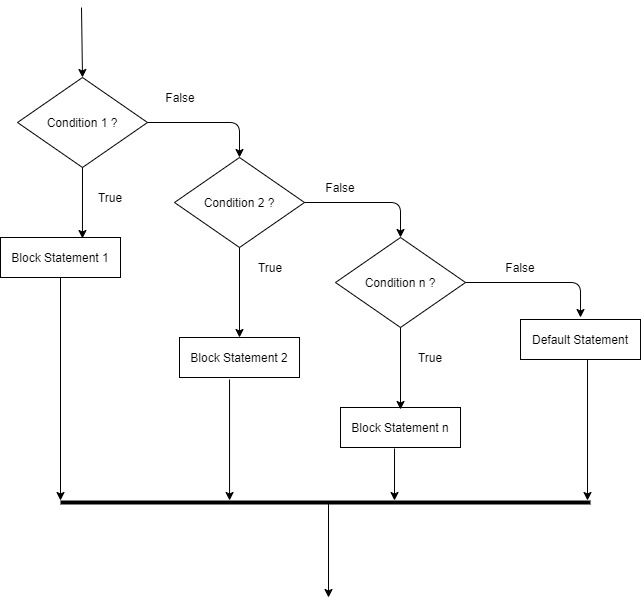
Example,
mark = int(input("Enter your mark"))
if(mark >= 90):
print("Grade: O")
elif(mark >= 80):
print("Grade: A+")
elif(mark >= 70):
print("Grade: A")
elif(mark >= 60):
print("Grade: B+")
elif(mark >= 50):
print("Grade: C")
elif(mark >= 45):
print("Grade: D")
else:
print("Fail")
Output
Output 1:
Enter your mark 75
Grade: A
Output 2:
Enter your mark 30
Fail
Nested if.. else
- One conditional can also be nested within another
- Any number of condition can be nested inside one another
- In this, if the condition is true it checks another condition. If both the conditions are true, then one set of statements are executed
Syntax
if(condition):
if(condition 1):
Statement 1
else:
Statement 2
else:
Statement 3
Flowchart
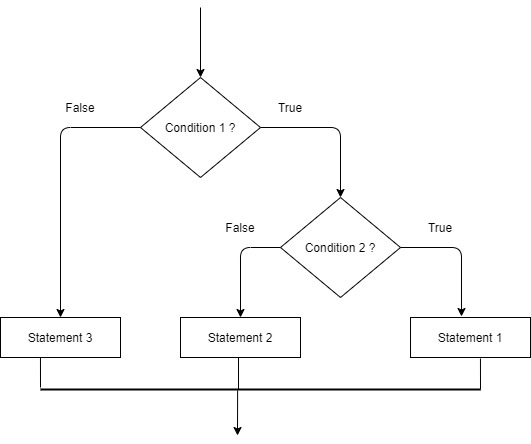
Example
num1 = int(input("Enter first number :"))
num2 = int(input("Enter second number:"))
num3 = int(input("Enter third number:"))
if(num1 > num2):
if(num1 > num3):
print("The greatest number is :",num1)
else:
print("The greatest number is :", num3)
else:
if(num2 > num3):
print("The greatest number is:",num2)
else:
print("The greatest number is :", num3)
Output
Enter first number :5
Enter second number:6
Enter third number:2
The greatest number is: 6
Views: 0