Table of Contents
show
State
Transition from one process to another process under specified condition with in a time is called state.
Loops
while loop and for loop
Loop Control statements
break, continue, pass
while loop
- while loop in python is used to repeatedly executes set of statement as long as a given condition is true
- In while loop, test expression is checked first. The body of the loop is entered only if the test expression is true. After one iteration, the test expression is checked again. This process continuous until the test_expression evaluates to False
- In python, the body of the while loop is determined through indentation
- The statements inside the while starts with indentation and the first unindented line marks the end
Syntax
while(condition):
body of while loop
increment
Flowchart
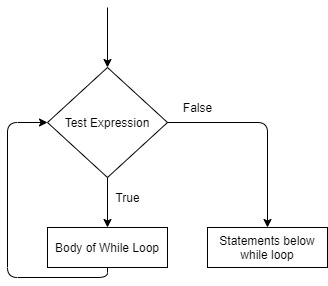
Example,
n=eval(input("enter the number"))
i = 1
sum = 0
while(i <= n):
sum = sum + i
i = i+1
print("sum of",n,"numbers = ", sum)
Output
enter the number 5
sum of 5 numbers = 15
else in while
When else statement is used with while loop, the else part will be executed when the condition become false
i = 1
while(i <= 5):
print(i,end=" ")
i=i+1
else:
print("Number greater than 5")
Output,
1 2 3 4 5 Number greater than 5
for
for in sequence: loop is used to iterate over a sequence (list, tuple, string). Iterating a sequence is called traversal
Syntax
for i in sequence:
print (i)
Example,
For loop in string
for i in "python":
print (i)
Output,
p
y
t
h
o
n
For loop in list
for i in [2,3,4,5]:
print (i)
Output
2
3
4
5
For loop in tuple
for i in (2,3,4):
print(i)
Output
2
3
4
for in range
Sequence of numbers can be generate using range( ) function. range(10) will generate numbers from 0 to 9.Range function is defined with start, stop and step size as range(start, stop, step) . step will be equal to 1 if not provided.
Syntax,
for i in range (start, stop, step):
body of for loop
Example,
for i in range(1,5,1):
print(i)
Flowchart
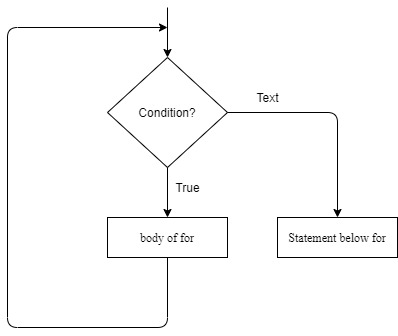
else in for
- If else statement is used in for loop, the else statement is executed when the loop has reached the limit
- The statements inside for loop and statements inside else will also execute
Example
for i in range(1,6):
print(i,end=" ")
else:
print("Number equal to 6")
Output,
1 2 3 4 5 Number equal to 6
Views: 0