One dimensional array
Omitting the start index starts the slice from the index 0.
Meaning, A[: stop] is equivalent to A[0:stop]
Omitting the stop index extends the slice to the end of the array.
Meaning A[start:] is equivalent ot A[start:len(A)]
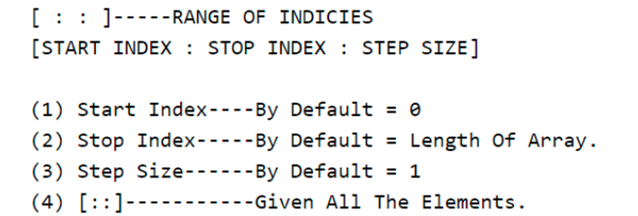
Two dimensional array
In 2D array, the elements at each index are no longer scalars, but rather one-dimensional array
np.array([[10,20,30], [40,50,60], [70,80,90]])
[40,50,60] – Index 1
[70,80,90] – Index 2
Multidimensional arrays can have one index per axis. These indices are given in a tuple separated by commas
Slice – selects a range of elements along an axis
Multiple slices can be passed as like multiple index
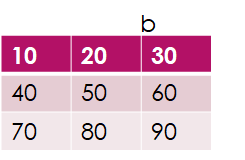
b[:2] – Slicing along row ( 2 exclusive)
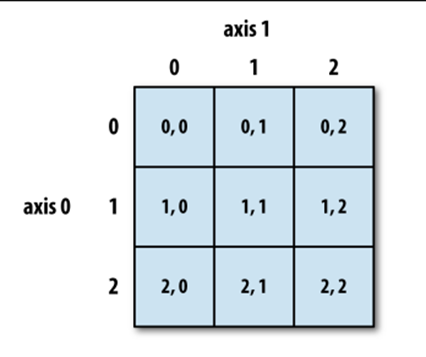
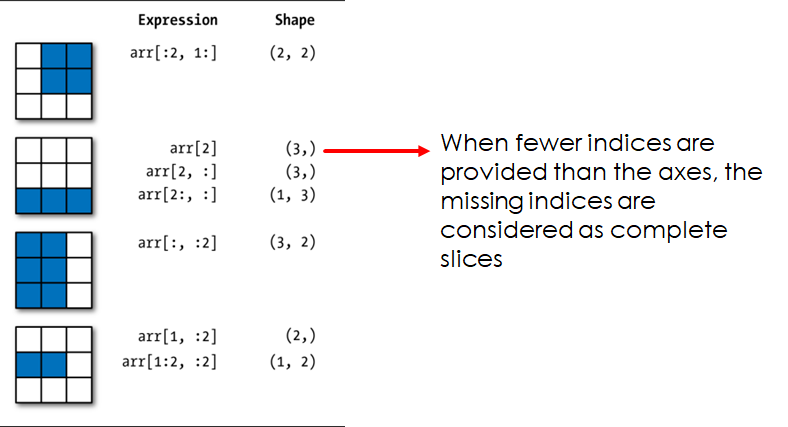
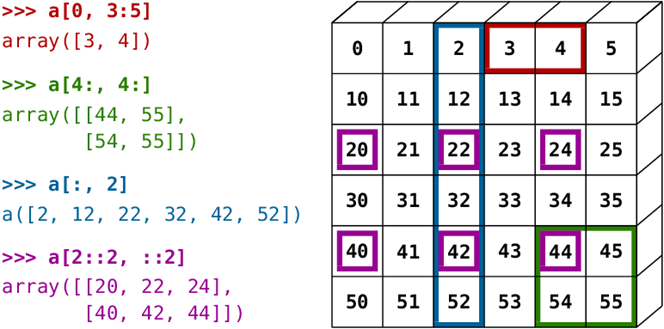
fromfunction( )
construct an array by executing a function over each coordinate and the resulting array,
def f(x,y):
return 10*x+y
b1 = np.fromfunction(f,(5,4),dtype=int)
b1
array([[ 0, 1, 2, 3],
[10, 11, 12, 13],
[20, 21, 22, 23],
[30, 31, 32, 33],
[40, 41, 42, 43]])
#getting particular element
print("b1[2,3]=",b1[2,3])
#Slicing and indexig
print("b1[0:3,2]=",b1[0:3,2])
print("b1[:,1]=",b1[:,1])#first column
print("b1[1:3,:]=",b1[1:3,:])
b1[2,3]= 23
b1[0:3,2]= [ 2 12 22]
b1[:,1]= [ 1 11 21 31 41]
b1[1:3,:]= [[10 11 12 13]
[20 21 22 23]]
Multi Dimensional Array
In Multidimensional arrays, If later indices are omitted, the returned object will be a lower dimensional ndarray consisting of all the data along the higher dimensions
Boolean Indexing
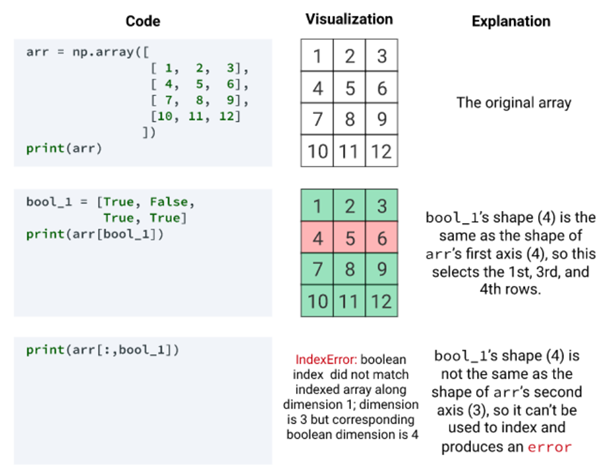
Passing Boolean array as index
narr1 = np.array([10,20,30,40])
narr2 = np.array([False,True,False, True])
print("Selected elements:",narr1[narr2])
Selected elements: [20 40]
Selecting even numbers based on Boolean array
ba1=np.empty(10)
for i in range(10):
ba1[i] = i
print(ba1)
ba2 = (ba1 % 2 == 0)
print(ba2)
print("even numbers:",ba1[ba2])
[0. 1. 2. 3. 4. 5. 6. 7. 8. 9.]
[ True False True False True False True False True False]
even numbers: [0. 2. 4. 6. 8.]
data
ba3 = np.array(['ram','abi','ravi','ram','ravi'])
data = np.random.randn(5,4)
print(data)
ba4 = (ba3 =='ram')
print("ba4",ba4)
#search for specific name
print(data [ ba3 == 'ram']) # boolean array can be passed when indexing the array:
[[-1.15594765 1.30522028 -2.49459926 0.39999895]
[ 1.47418789 -0.1766457 0.39876725 -0.56476218]
[ 1.55699037 1.30027161 -1.21973007 0.36258873]
[ 0.03846156 0.44934939 -0.25101183 2.13427514]
[-0.38013597 -0.36425022 0.42257762 -0.01005772]]
ba4 [ True False False True False]
[[-1.15594765 1.30522028 -2.49459926 0.39999895]
[ 0.03846156 0.44934939 -0.25101183 2.13427514]]

#boolean array must be of the same length as the axis it’s indexing. even mix and match boolean arrays with slices or integers
print("data[ba3 == 'abi',2]",data[ba3 == 'abi',2])
print("data[ba3 == 'abi',2:]",data[ba3 == 'abi',2:])
data[ba3 == 'abi',2] [0.39876725]
data[ba3 == 'abi',2:] [[ 0.39876725 -0.56476218]]
Select everything but not ram
#Select everything but not ram
print("(ba3 != 'ram') = ",ba3 != 'ram')
(ba3 != 'ram') = [False True True False True]
Selecting two of the three names to combine multiple boolean conditions, use boolean arithmetic operators like & (and) and | (or)
#Selecting two of the three names to combine multiple boolean conditions, use boolean arithmetic operators like & (and) and | (or):
mask = ( ba3 == 'ram') | (ba3 == 'abi')
print("mask=",mask)
mask= [ True True False True False]
Setting values with boolean array
set all of the negative values in data to 0
data[data<0] =0
print("Now data=",data)
Now data= [[0. 1.30522028 0. 0.39999895]
[1.47418789 0. 0.39876725 0. ]
[1.55699037 1.30027161 0. 0.36258873]
[0.03846156 0.44934939 0. 2.13427514]
[0. 0. 0.42257762 0. ]]
Setting whole rows or columns using 1D boolean array
data[ba3 != 'abi'] = 1
print(data)
[[1. 1. 1. 1. ]
[1.47418789 0. 0.39876725 0. ]
[1. 1. 1. 1. ]
[1. 1. 1. 1. ]
[1. 1. 1. 1. ]]
Fancy Indexing
Base Array:
for i in range(8):
arr[i] = i
print(arr)
[[0. 0. 0. 0.]
[1. 1. 1. 1.]
[2. 2. 2. 2.]
[3. 3. 3. 3.]
[4. 4. 4. 4.]
[5. 5. 5. 5.]
[6. 6. 6. 6.]
[7. 7. 7. 7.]]
Select a subset of rows in particular pass a list or ndarray of integers in desired order (arr)
print(arr[[4,3,1,6]])
Output
[[4. 4. 4. 4.]
[3. 3. 3. 3.]
[1. 1. 1. 1.]
[6. 6. 6. 6.]]
Use negative indexing to select from the end
print(arr[[-2,-3,-1,-5]])
Output
[[6. 6. 6. 6.]
[5. 5. 5. 5.]
[7. 7. 7. 7.]
[3. 3. 3. 3.]]
Passing multiple index arrays
#Passing multiple index arrays
arr1 = np.arange(32).reshape((8,4))
print(arr1)
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]
[16 17 18 19]
[20 21 22 23]
[24 25 26 27]
[28 29 30 31]]
Selects a 1D array of elements corresponding to each tuple of indices
#Selects a 1D array of elements corresponding to each tuple of indices
print(arr1[ [1,4,2,6],[2,3,1,0] ])
# the elements at (1,2) , (4,3), (2,1) and (6,0) are selected
Output
[ 6 19 9 24]
To select the rectangular region formed by selecting a subset of matrix’s row and column
print(arr1[ [1,4,2,6] ] [:,[2,3,1,0]] )
the elements at the position (1,2); (1,3);(1,1);(1,0) are selected for first row and so on
Output
[[ 6 7 5 4]
[18 19 17 16]
[10 11 9 8]
[26 27 25 24]]
Function converts two 1D integer arrays to an indexer that selects the square region
np_ix_ function converts two 1D integer arrays to an indexer that selects the square region
print(arr1[np.ix_( [1,4,2,6], [2,3,1,0] )])
the elements at the position (1,2); (1,3);(1,1);(1,0) are selected for first row and so on
Output
[[ 6 7 5 4]
[18 19 17 16]
[10 11 9 8]
[26 27 25 24]]
Views: 0