Array Creation
Arrays are displayed as a list or list of lists and can be created through list as well.
When creating an array we pass in a list as an argument in numpy array
a = np.array([1,2,3])
To print the number of dimensions of a array using the ndim attribute
a.ndim
We pass in a list of list in numpy array, to create a multi dimensional array
b= np.array( ( [1,2,3], [4,5,6]) )
The length of the array can be printed by calling the method shape
b.shape
To check the type of items in the array
b.dtype
Besides integers, floats are also accepted in numpy arrays
C = np.array ([2.2, 5, 1.1])
On seeing the data type of C,
C.dtype.name
Numpy automatically converts all integers into floating point values
Numpy: all the elements in the array must be same type
Array type can be specified explicitly at creation time
C = np.aray ([1,2],[3,4], dtype = complex)
- Often, the elements of an array are originally unknown, but its size is known
- Numpy offers several function to create arrays with initial placeholder content
- These minimize the necessary of growing arrays, an expensive operation
- zeros() – create an array of full of zeros
- ones() – create an array full of ones
- empty( ) – creates an array whose initial content is random
- By default, the dtype of the created array is float64
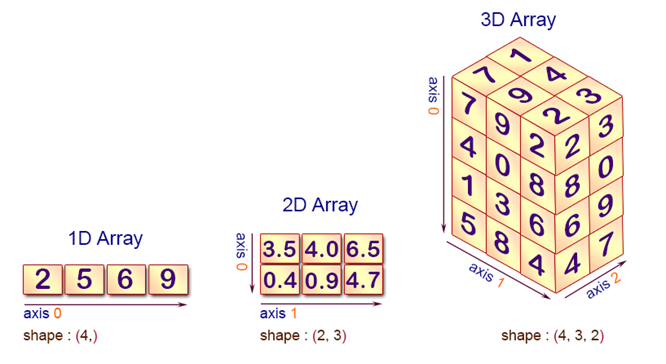
Array Creation functions
Numpy Array
Creates an array
numpy.array(object, dtype=None, *, copy=True, order='K', subok=False, ndmin=0, like=None)
Parameters
Object: An array, any object exposing the array interface, an object whose_array_method returns an array, or any (nested) sequence.
dtype: data type, optional (default data type is float)
copy: bool, optional. If true (optional) then, the object is copied
order: {‘K’, or ‘A’ or ‘C’ or ‘F’} optional
subok: bool, optional
ndim: int (optional) – specifies the minimum number of dimensions that the resulting array should have
like: Reference object to allow the creation of arrays which are not numpy arrays
Returns
out: ndarray
a) Create array using numpy.array
# Creating array by passing a list
a = np.array([1,2,3])
print("array by passing list:", a)
array by passing list: [1 2 3]
#upcasting
a1 = np.array([10.0,4,5])
print("upcasting:",a1)
upcasting: [10. 4. 5.]
b) Print number of dimensions of array
# to print number of dimensions of a array
a.ndim
1
c) Creating multidimensional array using list of list
#Creating multidimensional array using list of list
# np.array ( ([1,2,3],[4,5,6]) ) is also a sequence of sequence
b = np.array([[1,2,3],[4,5,6]])
b
array([[1, 2, 3],
[4, 5, 6]])
numpy.asarray
Convert the input to an array
numpy.asarray(a, dtype=None, order=None, *, like=None)
Parameters
a: array like; Input data in any form that can be converted to an array. It includes lists, list of tuples, tuples, tuples of tuples, tuples of list and ndarrays
dtype: data type, optional (default data type is float)
order: {‘K’, or ‘A’ or ‘C’ or ‘F’} optional
like: Reference object to allow the creation of arrays which are not numpy arrays
Returns
out : ndarray
using numpy.asarray
asa = [10,40,5]
asa1 = np.asarray(asa)
print("Using asarray:",asa1)
Using asarray: [10 40 5]
Changing the data type
asa = [10,40,5]
asa2 = np.asarray(asa,dtype=float)
print("Using asarray:",asa2)
Using asarray: [10. 40. 5.]
numpy.arange
Returns an array with evenly spaced elements as per the interval
numpy.arrange(start, stop, step, dtype)
Parameters
Start: (optional) start of interval range. By default start is 0; (inclusive)
stop: end of interval range
step: (optional) step size of interval, default is 1l it represents distance between adjacent elements
dtype: type of output array
Returns
out: nd array
Creating a sequence of numbers in an array with arange()
#generating only even numbers; first argument inclusive ; second argument - exclusive; third argument-difference between each consecutive numbers
F= np.arange(10,50,2)
print("F:",F)
#third argument can be floating point
G = np.arange(0,2,0.3)
print("G:",G)
F: [10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48]
G: [0. 0.3 0.6 0.9 1.2 1.5 1.8]
H0 = np.arange(10)
print("Generating float array:",H0)
H = np.arange(10, dtype = float)
print("Generating float array:",H)
Generating float array: [0 1 2 3 4 5 6 7 8 9]
Generating float array: [0. 1. 2. 3. 4. 5. 6. 7. 8. 9.]
numpy.ones
Returns a new array of a given shape and fill with ones
Syntax
numpy.ones ( shape, dtype = None, order=‘C’)
Parameters
Shape: integer or sequence of integers
dtype: optional
Order: C or F (optional)
Returns:
ndarray of ones having given shape, order and datatype
When elements of an array are not known but its size is known
# Creating an array full of ones
one_o = np.ones(1)
print("one_o",one_o)
f=np.ones((2,3)) #pass sequence
print("ones with shape:",f)
f1 = np.ones((3,2),dtype=int)
print("ones with shape and dtype:", f1)
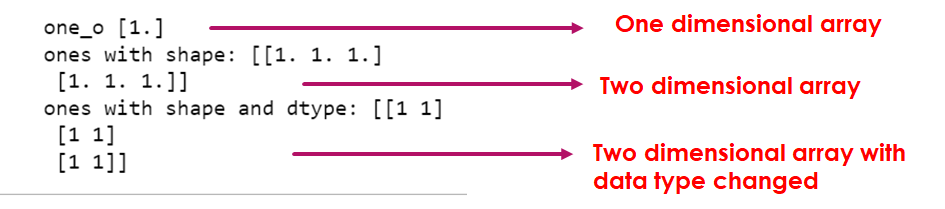
numpy.ones_like
Return an array of ones with the same shape and type as a given array
numpy.ones_like(a, dtype=None, order='K', subok=True, shape=None)
Parameter
a: array
dtype : data – type (optional)
order: {‘C’,’F’,’A’ or ‘K’} (optional)
subok : bool (optional)
shape : int or sequence of ints (optional)
Returns
out: ndarray
Array of ones with the same shape and type as a
‘F’ means to flatten in column-major (Fortran- style) order.
‘A’ means to flatten in column-major order if a is Fortran contiguous in memory, row-major order otherwise.
‘K’ means to flatten a in the order the elements occur in memory. The default is ‘C’.
myl = [1,2,3,4]
mya = np.array(myl)
myb = np.ones_like(mya)
print("Array",myb)
myb1 = np.ones_like(mya,float,'F',True,(2,2))
print ("Array in Column major order:",myb1)

numpy.zeros
Returns a new array of given shape and type, with zeros.
Syntax
numpy.zeros(shape, dtype=None, order=‘C’)
Parameters
shape: integer or sequence of integers
dtype: optional
Order: C or F (optional)
Returns
ndarray of ones having given shape, order and datatype
#creating an array of full zeros
one_e = np.zeros(10)
print("one_e:",one_e)
e = np.zeros((2,3)) #pass sequence
print("zeros with shape",e)
e1 = np.zeros((3,4),dtype=int)
print("zeros with shape and type",e1)

numpy.zeros_like
Return an array of zeros with the same shape and type as a given array
numpy. zeros_like(a, dtype=None, order='K', subok=True, shape=None)
Parameter
a: array
dtype: data – type (optional)
order: {‘C’,’F’,’A’ or ‘K’} (optional)
subok: bool (optional)
shape: int or sequence of ints (optional)
Returns
out: ndarray
Array of ones with the same shape and type as a
myc0 = [[1,2],[4,5]]
myc = np.zeros_like(myc0)
print("Two dimensional zero array:",myc)
#changing shape
myc1 = np.zeros_like(myc0,float,'F',True,1) # when subok attribute is set not passed, then shape of the array remains same as myc0
print("The dimension changed:",myc1)

numpy.empty
Returns a new array of given shape and type with random values
numpy.empty(shape, dtype=float, order=‘C’)
Parameter
shape : int or sequence of ints (optional)
dtype : optional ( float by default )
order : C or F
Returns
out: ndarray
Array of random values
#using empty function
rand_arra1 = np.empty((1,3)) #pass a sequence
print("Random array:",rand_arra1)
rand_arra2 = np.empty((1,3),dtype=int)
print("Random array with integer values", rand_arra2)
rand_arra3 = np.empty(2,dtype=int) # ----------------> 1 row with two column
print("Random array with integer values", rand_arra3)

numpy.empty_like
Return an array of random values with the same shape and type as a given array.
numpy.empty_like(a, dtype=None, order='K', subok=True, shape=None)
Parameter
a: array
dtype : data – type (optional)
order: {‘C’,’F’,’A’ or ‘K’} (optional)
subok : bool (optional) shape : int or sequence of ints (optional)
Returns
out: ndarray
Array of random values with the same shape and type as a
mye0 = [[1,2],[4,5]]
mye1 = np.empty_like(mye0)
print("Two dimensional random array:",mye1)
#changing shape
mye2 = np.empty_like(mye0,float,'F',True,1) # when subok attribute is set not passed, then shape of the array remains same as myc0
print("The dimension changed:",mye2)

numpy.eye
Returns a 2-D array with 1’s as the diagonal and 0’s elsewhere
The diagonal can be main, upper or lower depending on the optional parameter k
Positive k is for upper diagonal, a negative k is for lower and a 0 k is for main diagonal (default)
numpy.eye(R, C = None, k = 0, dtype = type <‘float’>)Â
Parameters:
R : number of rows
C : (optional) number of columns; by default M=N
dtype : optional; by default float
Return:
2- D array
Using eye to create a array
myi1 = np.eye(4)
print("Creating array with only row R:",myi1)
myi2 = np.eye(4,5)
print("Creating array with R and C",myi2)
myi3 = np.eye(4,4,-1)
print("Creating array with R and C",myi3)
myi4 = np.eye(4,4,1)
print("Creating array with R and C",myi4)
myi5 = np.eye(4,4,2)
print("Creating array with R and C",myi5)
myi6 = np.eye(4,4,-2)
print("Creating array with R and C",myi6)
Creating array with only row R: [[1. 0. 0. 0.]
[0. 1. 0. 0.]
[0. 0. 1. 0.]
[0. 0. 0. 1.]]
Creating array with R and C [[1. 0. 0. 0. 0.]
[0. 1. 0. 0. 0.]
[0. 0. 1. 0. 0.]
[0. 0. 0. 1. 0.]]
Creating array with R and C [[0. 0. 0. 0.]
[1. 0. 0. 0.]
[0. 1. 0. 0.]
[0. 0. 1. 0.]]
Creating array with R and C [[0. 1. 0. 0.]
[0. 0. 1. 0.]
[0. 0. 0. 1.]
[0. 0. 0. 0.]]
Creating array with R and C [[0. 0. 1. 0.]
[0. 0. 0. 1.]
[0. 0. 0. 0.]
[0. 0. 0. 0.]]
Creating array with R and C [[0. 0. 0. 0.]
[0. 0. 0. 0.]
[1. 0. 0. 0.]
[0. 1. 0. 0.]]
numpy.identity
Returns the identity array
numpy.identity(n, dtype = None)
Return a identity matrix i.e. a square matrix with ones on the main diagonal.
Parameters:
n : [int] Dimension n x n of output array
dtype : [optional, float(by Default)] Data type of returned array.
Optional data type float
myi = np.identity(2)
myi
array([[1., 0.],
[0., 1.]])
data type int
nw1 = np.identity(4, dtype=int)
nw1
array([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]])
Array Creation
Create two arrays, both with same shape but with different filler values
Either the parameters 2,3 has to be passed as (2,3) or [2,3]
D = np.zeros((2,3))
E = np.ones((2,3))
# to change the type to int
np.ones((2,3), dtype = int)
Generate an array with random numbers
np.random.rand(2,3)
np.empty((2,3))
Create a sequence of numbers in an array with arrange ( ) function
The first argument is the starting bound and the second argument is the ending bound and the third argument is the difference between each consecutive numbers
Create an array of every even number from ten (inclusive) to fifty (exclusive)
F = np.arange(10, 50, 2)
The third argument can be floating point number also,
F = np.arange(0, 2, 0.3)
Genearate sequence of floats
Use linspace() function,
- The third argument is not the difference between two numbers, but the total number of items you want to generate
- Both the first argument and second argument are inclusive
np.linspace(0,2,15)
np.nandomrand()
np.random.randint()
randint() method returns an integer number in the specified range
Syntax
random.randint(low,high=None,size=None, dtype = int)
np.random.randn()
Creates an array of specified shape and fills it with random values as per standard normal distribution.
Syntax
numpy.random.rand(d0,d1,d2,…,dn)
d0,d1,d2,..,dn represents the number of random numbers to generate
Printing Arrays
- One dimensional arrays are printed as rows
- Bi dimensional arrays are printed as matrices
- Tri dimensional arrays are printed as lists of matrices
a = np.arrange(6) # prints 1 dimensional array
B = np.arrange(12).reshape(4,3) # prints 2-d array with 2 rows and 3 columns
C = np.arrange(24).reshape(2,3,4) # prints 3-d array with 2 orows each of three columns and 4 values in each row
Impact of reshape
If an array is too large to be printed, numpy automatically skips the central part of the array and only prints the corners
reshape – gives a new shape to an array without changing its data
Syntax
numpy.reshape(a, newshape, order)
A – an array
Newshape – int or tuple of int
Order: {‘C’, ‘F’, ‘A’}
Impact of reshape
np.arrange(10000)
np.arrange(10000).reshape((100,100))
Data types
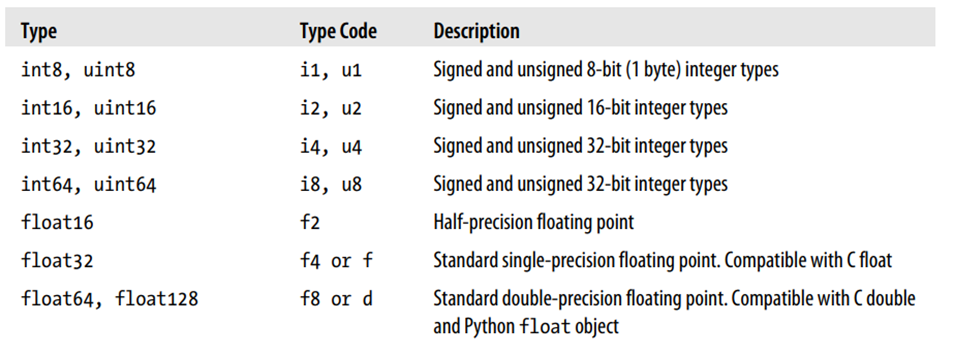
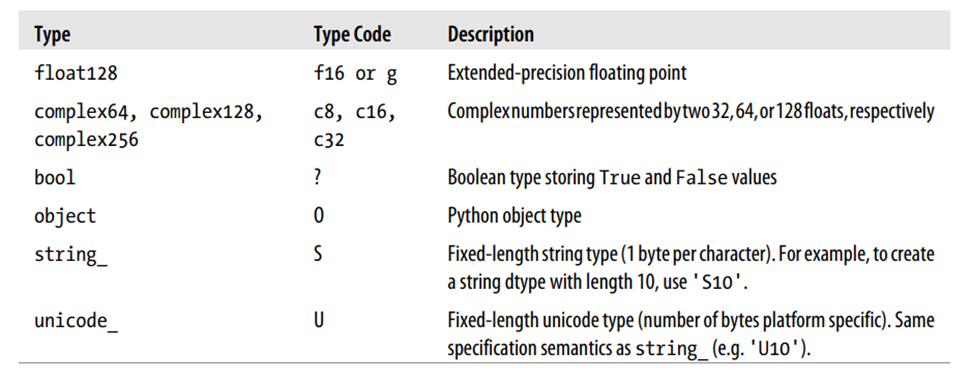
Views: 0