Table of Contents
show
Definition
Image Blending is a Digital Image processing technique used to combine two or more images to create a composite image.
The goal is to merge the images so that the boundaries between them are not easily noticeable
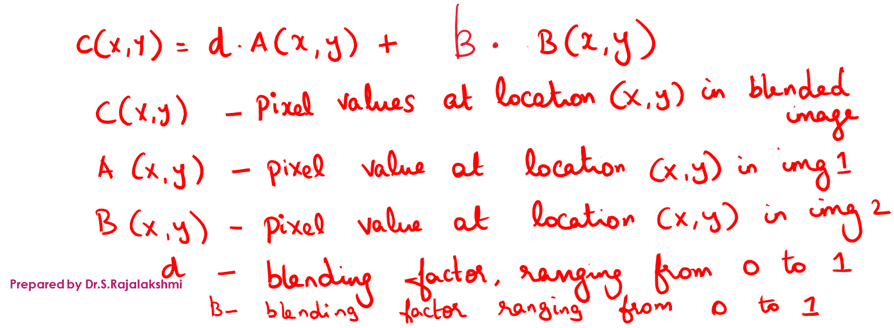
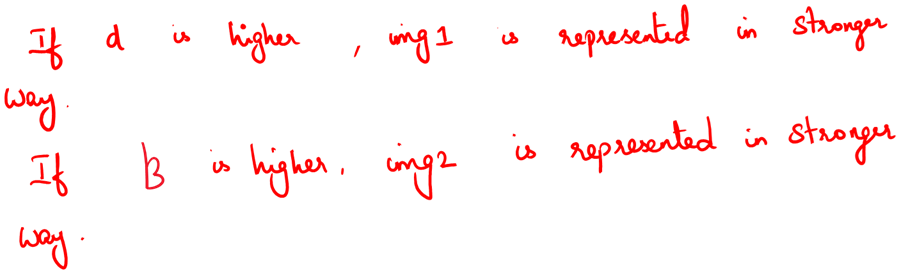
Use of addWeighted()
addWeighted( ) – works only for images of same size
Syntax
cv2.addWeighted(src1, alpha, src2, beta, gamma, dst=None, dtype=None)
- src1: First input array or image.
- alpha: Weight of the first array/image.
- src2: Second input array or image.
- beta: Weight of the second array/image.
- gamma: Scalar added to each sum. Usually, it’s set to 0 for simple blending.
- dst: Optional output array that has the same size and type as src1 and src2. If not provided, a new array will be created.
- dtype: Optional data type of the output array. If not provided, the data type of src1 is used.
Bitwise operations
cv2.bitwise_not(src, dst=None, mask=None)
- src (required): The input array (image) on which the bitwise NOT operation will be performed. It should be of type CV_8U.
- dst (optional): The output array that will store the result of the operation. It should be of the same size and type as src. If not specified, the result is returned.
- mask (optional): An optional mask array of the same size as src that specifies which elements of the output array are changed. This is useful if you only want to invert specific parts of the input array.
cv2.bitwise_or(src1, src2, dst=None, mask=None)
- src1 (required): The first input array (image). It must have the same size and type as src2.
- src2 (required): The second input array (image). It must have the same size and type as src1.
- dst (optional): The output array where the result is stored. If not provided, the function will create and return the output array.
- mask (optional): An optional mask array. This is used to specify which elements of the result array are modified. The mask should be of the same size as src1 and src2. Pixels in the result are updated only where the mask has non-zero values.
cv2.bitwise_and(src1, src2, dst=None, mask=None)
- src1 (required): The first input array (image). It must have the same size and type as src2.
- src2 (required): The second input array (image). It must have the same size and type as src1.dst (optional): The output array where the result is stored. If not provided, the function will create and return the output array.
- mask (optional): An optional mask array. This specifies which elements of the result array are modified. The mask should be of the same size as src1 and src2. Pixels in the result are updated only where the mask has non-zero values.
Creating array with full
The np.full function in NumPy is used to create a new array of a specified shape and fill it with a specific value.
numpy.full(shape, fill_value, dtype=None, order='C')
- shape (required): The shape of the new array.
- fill_value (required): The value with which to fill the array.
- dtype (optional): The desired data type of the array. If not specified, the data type is inferred from fill_value. For example, if fill_value is an integer, the default dtype will be np.int64 or np.int32, depending on your system.
- order (optional): Whether to store multidimensional data in C-contiguous (row-major) or F-contiguous (column-major) order in memory. The default is ‘C’.
Views: 1