Table of Contents
show
Resizing the image
cv2.resize(src, dsize, fx=0, fy=0, interpolation=cv2.INTER_LINEAR)
- src: The source image you want to resize.
- dsize: The desired size of the output image in the form (width, height). If this is set to (0, 0), the size is determined by fx and fy.
- fx: Scale factor along the horizontal axis. This is used when you don’t specify dsize.
- fy: Scale factor along the vertical axis. This is used when you don’t specify dsize.
- interpolation: The interpolation method to use. Some options include:
- cv2.INTER_NEAREST (nearest neighbor interpolation)
- cv2.INTER_LINEAR (bilinear interpolation, default) – considers 4 nearest neigbor
- cv2.INTER_CUBIC (bicubic interpolation) – considers 16 nearest pixels
- cv2.INTER_LANCZOS4 (Lanczos interpolation) – sin function based kernel
- cv2.INTER_AREA
Interpolation is the process of transferring image from one resolution to another without losing image quality
- Nearest Neigbor Interpolation – refer problems
- Bilinear Interpolation – refer problems
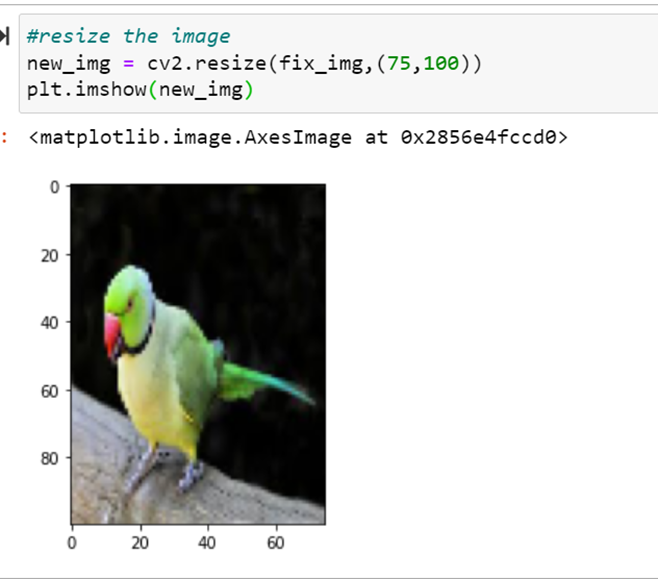
new_img = cv2.resize(fix_img,(75,100))
plt.imshow(new_img)
Good to Know
Resize the image
- Used to resize an image to a specified size
- This function allows you to scale the dimensions of an image up or down by providing the desired width and height.
Cropping the image
img(startY:endY, startX:endX)
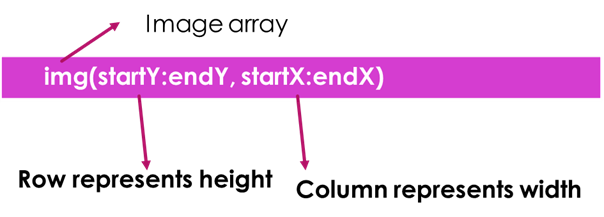
Example
image[50:200, 100:300]
- 50:200 refers to the pixel range along the Y-axis (height).
- 100:300 refers to the pixel range along the X-axis (width).
Opening the images in OpenCV (.py)
If the window is displayed for at least 1 milliseconds, and the user presses escape key ( number equivalent is 27), then the window will be closed.
import cv2
img = cv2.imread("Baboon.jpg")
while True:
#imshow()---> parameter 1 - name of the window ; parameter 2 -- image to be displayed
cv2.imshow("Baboon",img)
#If we have waited atleast 1 ms and we pressed Esc key
if cv2.waitKey(1) & 0xFF ==27:
break;
cv2.destroyAllWindows()
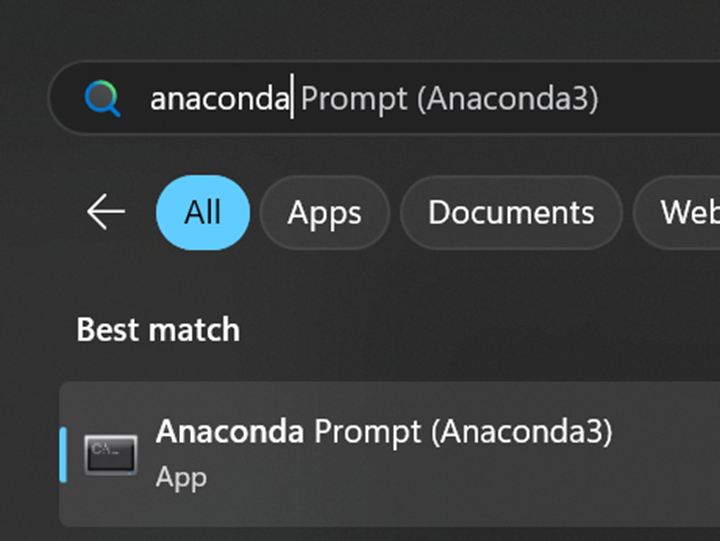
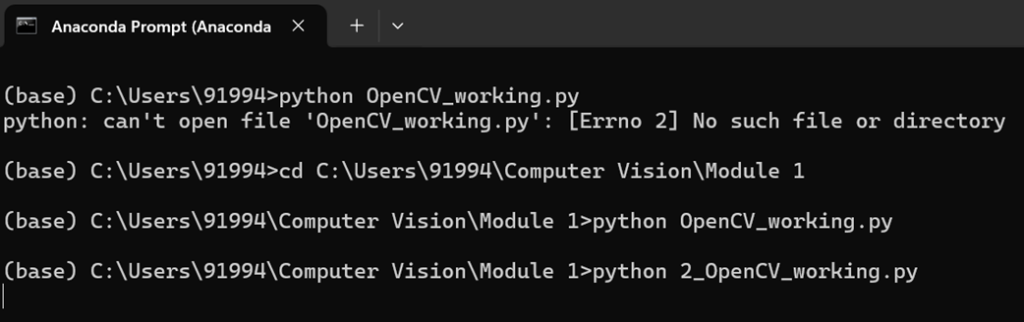
Views: 0