Table of Contents
show
Edges are places in an image where there is a sharp change in color or brightness.
- Edges: Lines or curves where the intensity (color or brightness) changes sharply.
Contours: Complete boundaries around an object, often formed by connecting edges.
Corners: Specific points where two or more edges meet, typically at sharp angles (like the corners of a square or triangle).
Example:
- Edges: Think of these as the sides of a room (walls).
- Contours: The full outline of the room (floor plan).
- Corners: The sharp points where two walls meet (corners of the room).
contours, hierarchy = cv2.findContours(image, mode, method)
- Image: The source binary image from which contours are to be found. This image should be in a single channel (grayscale) format. The original image will be modified
- mode:The contour retrieval mode, which determines how the contours are organized:
- cv2.RETR_EXTERNAL: Retrieves only the external contours.
- cv2.RETR_LIST: Retrieves all contours without any hierarchy.(No parent child relationship)
- cv2.RETR_CCOMP: Retrieves all contours and organizes them into a two-level hierarchy (external and internal). (The first level contains external contours, and the second level contains the contours of holes or internal contours.)
- cv2.RETR_TREE: Retrieves all contours and organizes them in a full hierarchy. (parent child relationship)
cv2.CHAIN_APPROX_SIMPLE:
- Compresses horizontal, vertical, and diagonal segments, keeping only their endpoints.
- This is useful for reducing the number of points.
- It removes all redundant points and compresses the contour, thereby saving memory.
- cv2.CHAIN_APPROX_NONE: Stores all the contour points without any approximation.
Returns:
- contours:A list of contours found in the image. Each contour is represented as a numpy array of (x, y) coordinates of the boundary points of the shape.
- hierarchy:An array that contains information about the hierarchy of the contours. It provides details about the relationship between the contours, such as parent-child relationships.
The hierarchy provides information about:
- Which contours are outer contours (external contours).
- Which contours are holes (internal contours) within those outer contours.
- The relationship (parent-child) between contours.
- [Next, Previous, First_Child, Parent]
Next:
- Definition: The index of the next contour at the same hierarchical level.
- Value: If this value is -1, there is no next contour at that level.
Previous:
- Definition: The index of the previous contour at the same hierarchical level.
- Value: If this value is -1, there is no previous contour at that level.
First Child:
- Definition: The index of the first child contour of the current contour. This indicates a nested contour.
- Value: If this value is -1, the contour does not have any child contours.
Parent:
- Definition: The index of the parent contour of the current contour. This indicates which contour contains the current contour.
- Value: If this value is -1, it means the contour is a top-level contour (not nested within any other contour).
RETR_LIST
- Parents and kids are equal under this rule, and they are just contours. ie they all belongs to same hierarchy level.
- 3rd and 4th term in hierarchy array is always -1.
RETR_EXTERNAL
- Retrieves only external contours
- ( Only the eldest in every family is taken care of. It doesn’t care about other members of the family
RETR_CCOMP
- Retrieves all the contours and arranges them to a 2-level hierarchy. ie external contours of the object (ie its boundary) are placed in hierarchy-1. And the contours of holes inside object (if any) is placed in hierarchy-2. If any object inside it, its contour is placed again in hierarchy-1 only. And its hole in hierarchy-2 and so on.
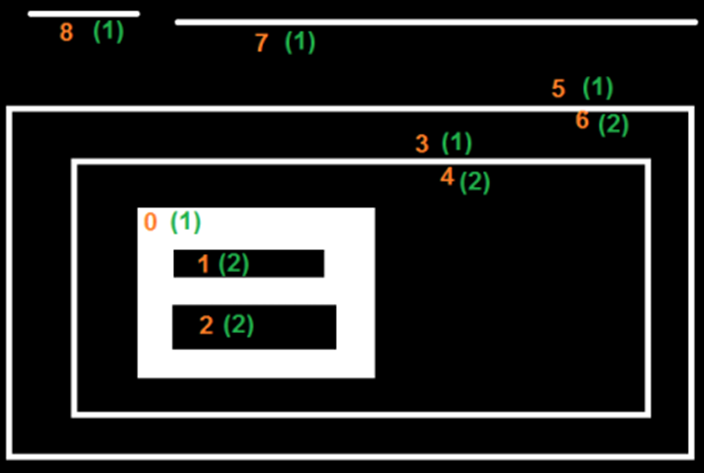
RETR_TREE
- It retrieves all the contours and creates a full family hierarchy list.Â
- It even tells, who is the grandpa, father, son, grandson and beyond
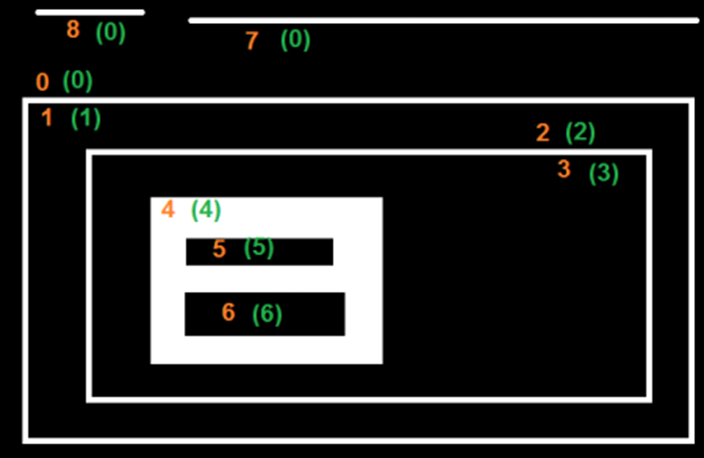
Since this is external contour, no parent, no previous, no next, no first child
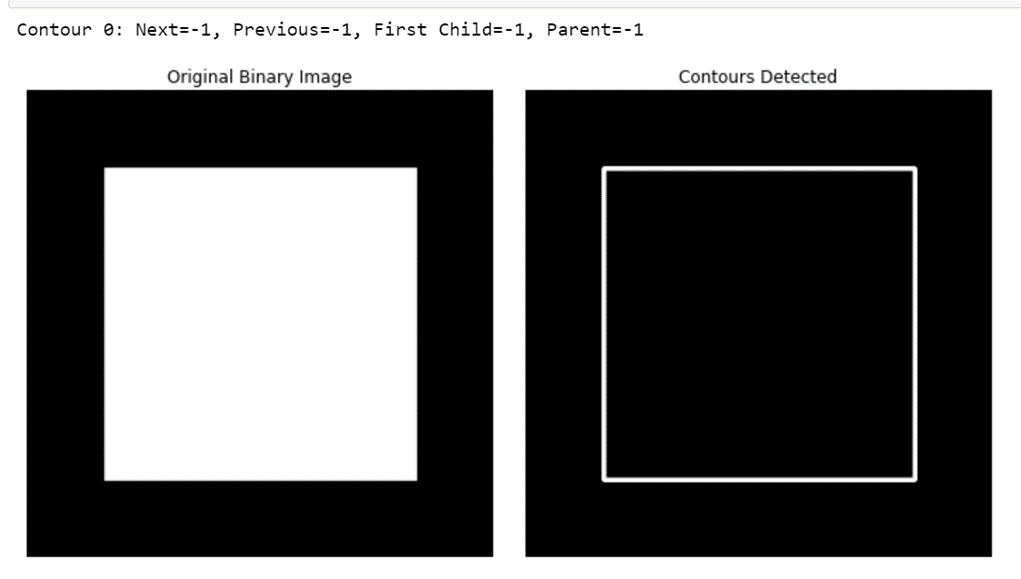
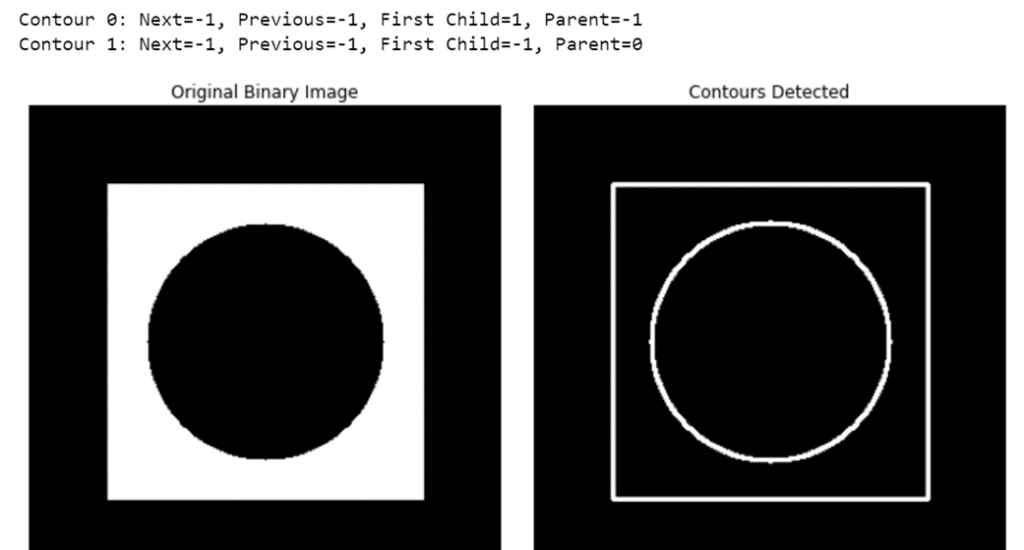
Identify Internal and External Contours
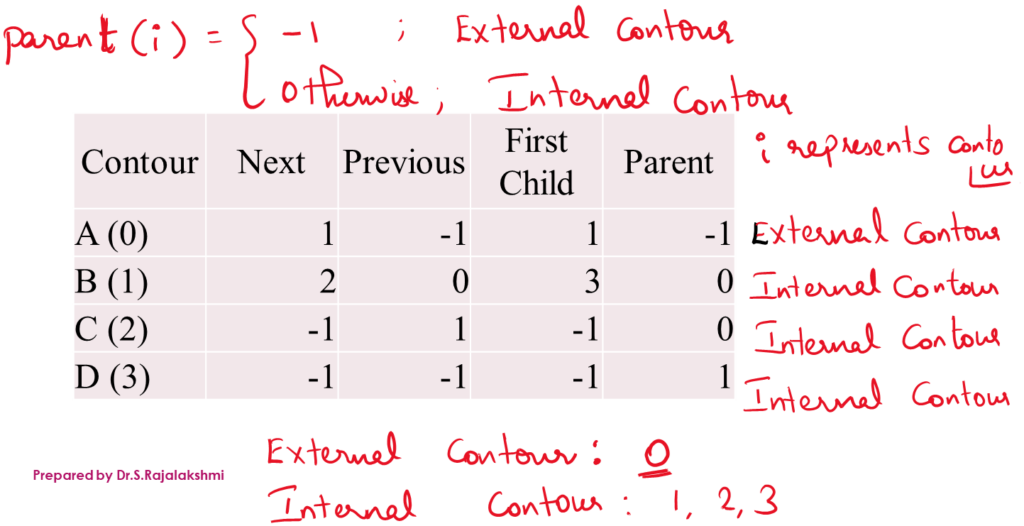
cv2.drawContours() function in OpenCV is used to draw contours on an image
- Image – the image on which the contours is drawn. Typically a grey scale image
- Contours – A list of contours that needs to draw. Contours are stored as list of points
- contourIdx – The index of the contours to be drawn from the list of contours
- color – The color of the contour.
- In grayscale images, 255 corresponds to white.
- For colored images, this can be a tuple like (255, 0, 0) for blue, (0, 255, 0) for green
- thickness – The thickness of the contour. -1 means that the contour will be filled in.
Views: 1