Table of Contents
show
The arguments that are given after the name of the program in the command line shell of the operating system are known as command line arguments. Various ways of using command line arguments are,
Using sys.argv
Using sys.argv
The sys module provides functions and variables used to manipulate different parts of the python runtime environment. This module provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter.
Example
sys.argv
It is a simplest list structure. Its main purposes are:
- It is a list of command line arguments
- len(sys.argv) provides number of command line arguments
- sys.argv[0] is the name of the current python script
Example
argc (ARGument Count)
- It is int and stores number of command-line arguments passed by the user including the name of the program. So, if we pass a value to a program, value of argc would be 2 (one for argument and one for program name)
- The value of argc should be non-negative.
argv(ARGument Vector)Â
- It is array of character pointers listing all the arguments.
- If argc is greater than zero,the array elements from argv[0] to argv[argc-1] will contain pointers to strings.
- Argv[0] is the name of the program , After that till argv[argc-1] every element is command -line arguments.
Example: To count number of words in a sentence
#include <stdio.h>
int main(int argc, char *argv[])
{
int i = 0;
/* If no sentence is given in the command line */
if (argc == 1)
{
printf("\n No sentence given on command line");
return 0;
}
else
{
printf("\nThe words in the sentence are:");
/*
* From argv[1] to argv[argc -1] calculate the number of arguments
*/
for (i = 1;i < argc;i++)
{
printf("\n%s", argv[i]);
}
printf("\n\nTotal number of words:");
printf(" %d", argc-1);
}
return 0;
}
Output
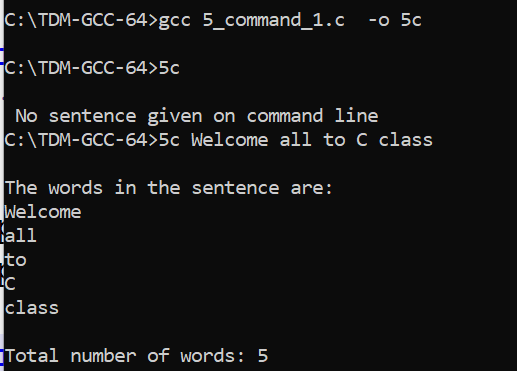
- sys.argv[0] – file name i.e 5c
- sys.argv[1] contains Welcome
- sys.argv[2] contains all
- sys.argv[3] contains to
- sys.argv[4] contains C
- sys.argv[5] contains class
Example: Generate Fibonacci series using command line arguments in C
#include <stdio.h>
/* Global Variable Declaration */
int first = 0;
int second = 1;
int third;
/* Function Prototype */
int rec_fibonacci(int);
int main(int argc, char *argv[])/* Command line Arguments*/
{
int number = atoi(argv[1]);
printf("%d\t%d", first, second); /* To print first and second number of fibonacci series */
rec_fibonacci(number);
printf("\n");
return 0;
}
/* Code to print fibonacci series using recursive function */
int rec_fibonacci(int num)
{
if (num == 2) /* To exit the function as the first two numbers are already printed */
{
return 0;
}
third = first + second;
printf("\t%d", third);
first = second;
second = third;
num--;
rec_fibonacci(num);
}
Output
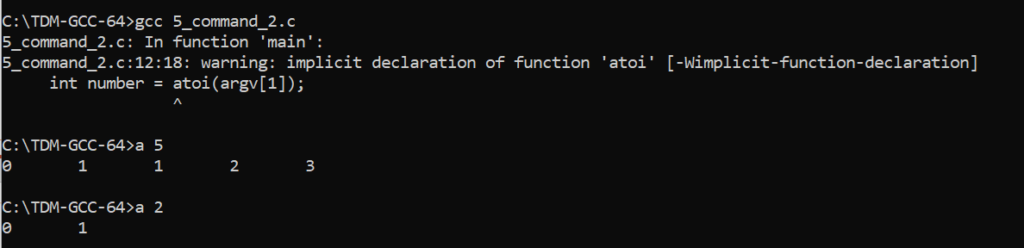
- sys.argv[0] = a // name of executable filename
- sys.argv[1] = 5
Views: 0