Table of Contents
show
The name of an array refers to the address of the first element of the array, i.e. an expression of array type decomposes to pointer type
int a[3] = {10,20,30};
Here,
a[0] = 10
a[1] = 20
a[2] =30
the same can be written as
*(a+0) = 10
*(a+1) = 20
*(a+2) = 30
printf(“Address of array = %u”, a)
The above statement prints the address of first element of array ‘a’
int *ptr = a; // Assigning address of a to ptr
*ptr = 10
*(ptr + 1) = 20
In general, *(ptr + i) gives the value of element in i th location.
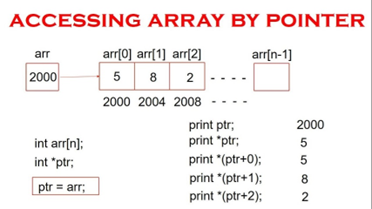
#include<stdio.h>
int main()
{
int arr[3] = {10,15,20};
printf("first element is at %p\n",arr);
printf("second element is at %p\n",arr+1);
printf("third element is at %p\n",arr+2);
return 0;
}
Output
first element is at 000000000062FE10
second element is at 000000000062FE14
third element is at 000000000062FE18
Program: C program to get the array element using pointer and print it using pointer
#include<stdio.h>
int main()
{
int a[100];
int n,i;
int *ptr;
printf("Enter size of array\n");
scanf("%d",&n);
ptr = a; // Initializing pointer with starting address of array a
// loop to read array elements
printf("Read Array Elements\n");
for(i = 0;i<n;i++)
{
scanf("%d",ptr);
ptr++;
}
//loop to print array elements
ptr = a;
printf("Array Elements are\n");
for(i=0;i<n;i++)
{
printf("%d\t",*ptr);
ptr++;
}
return 0;
}
Output
Enter size of array
5
Read Array Elements
3 4 2 1 6
Array Elements are
3 4 2 1 6
Program: C program to read array elements using pointer and print it in reverse order
#include<stdio.h>
int main()
{
int a[100];
int n,i;
int *ptr;
printf("Enter size of array\n");
scanf("%d",&n);
ptr = a; // Initializing pointer with starting address of array a
// loop to read array elements
printf("Read Array Elements\n");
for(i = 0;i<n;i++)
{
scanf("%d",ptr);
ptr++;
}
ptr = &a[n-1];
//loop to print array elements
printf("Array Elements are\n");
for(i=n-1;i>=0;i--)
{
printf("%d\t",*ptr);
ptr--;
}
return 0;
}
Output
Enter size of array
6
Read Array Elements
1 4 2 3 9 5
Array Elements are
5 9 3 2 4 1
The expression of the form E1[E2][E3] is automatically converted into an equivalent expression of the form *(*(E1+E2)+E3)
#include<Stdio.h>
int main()
{
int a[2][3] = {2,1,3,2,3,4};
printf("Using subscript operator\n");
printf("%d\t%d\t%d\n",a[0][0], a[0][1], a[0][2]);
printf("%d\t%d\t%d\n",a[1][0], a[1][1], a[1][2]);
printf("Using pointer \n");
printf("%d\t%d\t%d\n",*(*(a+0)+0),*(*(a+0)+1), *(*(a+0)+2) );
printf("%d\t%d\t%d\n",*(*(a+1)+0),*(*(a+1)+1), *(*(a+1)+2));
printf("Using mixed form \n");
printf("%d\t%d\t%d\n",*(a[0]+0),*(a[0]+1), *(a[0]+2) );
printf("%d\t%d\t%d\n",*(a[1]+0),*(a[1]+1), *(a[1]+2) );
return 0;
}
Output
Using subscript operator
2 1 3
2 3 4
Using pointer
2 1 3
2 3 4
Using mixed form
2 1 3
2 3 4
- The expression of the form E1[E2][E3] is automatically converted into an equivalent expression of the form *(*(E1+E2)+E3)
- The expression *(a+i) is equivalent to a[i].
- Hence the expression *(*(a+i)+j) is equivalent to *(a[i] +j) which is further equivalent to a[i][j]
- For a two dimensional array,
- When no subscript is used, the expression array name refers to the starting address of the first element(i.e. first row of the array
- When one subscript is used , the expression a[0] and a[1] represents the starting address of the array.
Views: 0