Sequential access
Sequential access files allow to read and write information sequentially starting from beginning of the file.
Sequential access file
#include <stdio.h>
#include <math.h>
int main(){
int i = 0, sum = 0, n = 0,avg = 0;
FILE *fin;
// Open file
fin = fopen("seq.txt", "r");
// Keep reading in integers (which are placed into "n") until end of file (EOF)
while(fscanf(fin, "%d", &n) != EOF)
{
// Add number to sum
sum += n;
// Increment counter for number of numbers read
i++;
}
// Average is sum of numbers divided by numbers read
avg = (sum / i);
// After the loop is done, show the average
printf("The average is %d.\n", avg);
fclose(fin);
return 0;
}
Output
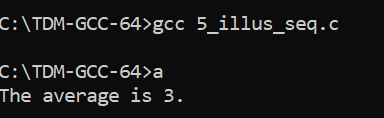
Random access
Random access files enable to read or write information anywhere in the file
Random access file
- Access individual records without searching through other records
- Instant access to records in a file
- Data can be inserted without destroying other data
- Data previously stored can be updated or deleted without overwriting
- Implemented using fixed length records
- Sequential files do not have fixed length records
Functions for random access
- fseek
- ftell
- rewind
fseek
sets the file position of the stream to the given offset.
Syntax
Parameters
stream − This is the pointer to a FILE object that identifies the stream.
offset − This is the number of bytes to offset from whence.
- 0 : sets the reference point at the beginning of the file
- 1: sets the reference point at the current file position
- 2: sets the reference point at the end of the file
whence − This is the position from where offset is added. It is specified by one of the following constants
- SEEK_SET – Beginning of file
- SEEK_CUR – Current position of the file pointer
- SEEK_END – End of file
Return Value
This function returns zero if successful, or else it returns a non-zero value.
ftell
Returns the current file position of the given stream.
Syntax
Parameters
stream − This is the pointer to a FILE object that identifies the stream.
Return Value
This function returns the current value of the position indicator. If an error occurs, -1L is returned, and the global variable errno is set to a positive value.
rewind
Sets the file position to the beginning of the file of the given stream.
Syntax
Parameters
stream − This is the pointer to a FILE object that identifies the stream.
Return Value
This function does not return any value.
Views: 0