Table of Contents
show
Transaction Processing System using random access files
#include<stdio.h>
#include<stdlib.h>
struct account
{
int number;
long amount;
char name[20];
};
void create()
{
FILE *fptr;
int i, n;
struct account acc;
if ((fptr = fopen("account.bin","wb")) == NULL)
{
printf("File Cannot Open!");
exit(1);
}
printf("Enter Total Number of Customers\n");
scanf("%d",&n);
for(i= 1; i <= n; i++)
{
acc.number=i;
printf("Enter Name of User %d : ", i);
scanf("%s",acc.name);
printf("Enter Initial Amount of User %d : ", i);
scanf("%ld",&acc.amount);
fwrite(&acc, sizeof(struct account), 1, fptr);
}
fclose(fptr);
}
void view()
{
FILE *fptr;
int n;
struct account acc;
if ((fptr = fopen("account.bin","rb")) == NULL)
{
printf("File Cannot Open!");
exit(1);
}
while(1)
{
printf("\nEnter Customer Number to be view : -1 to stop \n");
scanf("%d",&n);
if(n==-1)
break;
fseek(fptr,(n-1)*sizeof(struct account),0);
fread(&acc, sizeof(struct account), 1, fptr);
printf("Customer Number: %d\t Customer Name: %s \tBalance: %ld",acc.number,acc.name,acc.amount);
}
fclose(fptr);
}
void transfer()
{
int fromno,tono;
long tamount;
struct account acc,fromacc,toacc;
FILE *fptr;
if ((fptr = fopen("account.bin","rb+")) == NULL)
{
printf("File Cannot Open!");
exit(1);
}
printf("\nEnter From Account No:\n");
scanf("%d",&fromno);
printf("\nEnter To Account No:\n");
scanf("%d",&tono);
printf("\nEnter Amount to be transfer:");
scanf("%ld",&tamount);
fseek(fptr,(fromno-1)*sizeof(struct account),0);
fread(&fromacc, sizeof(struct account), 1, fptr);
fseek(fptr,(tono-1)*sizeof(struct account),0);
fread(&toacc, sizeof(struct account), 1, fptr);
fromacc.amount-=tamount;
toacc.amount+=tamount;
fseek(fptr,(fromno-1)*sizeof(struct account),0);
fwrite(&fromacc, sizeof(struct account), 1, fptr);
fseek(fptr,(tono-1)*sizeof(struct account),0);
fwrite(&toacc, sizeof(struct account), 1, fptr);
fclose(fptr);
}
int main()
{
int ch;
while(1)
{
printf("\n______MENU_______\n");
printf("1.Create Account\n2.View Account Detail \n3.Transfer Amount\n4.Exit");
printf("\nEnter Your Choice: ");
scanf("%d",&ch);
switch(ch)
{
case 1:
create();
break;
case 2:
view();
break;
case 3:
transfer();
break;
case 4:
exit(0);
}
}
return 0;
}
Output
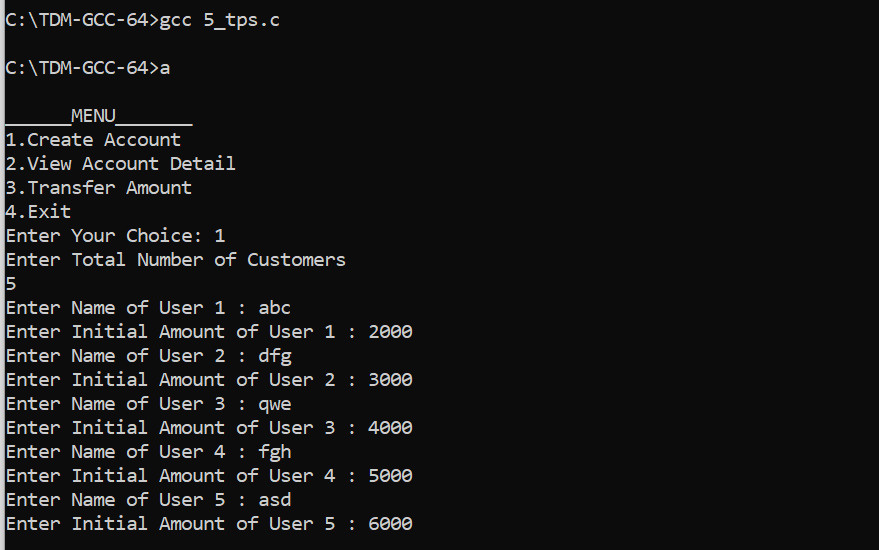
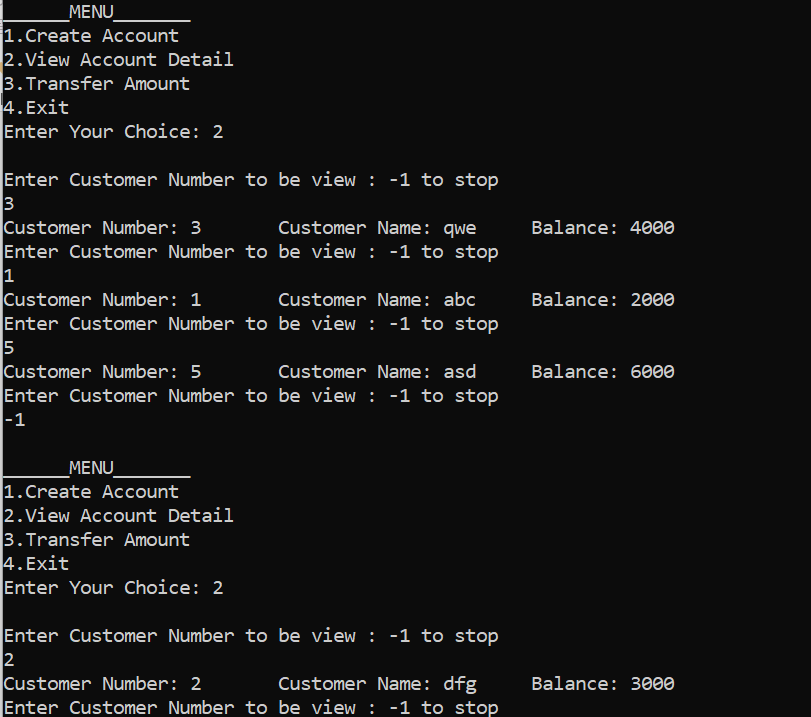
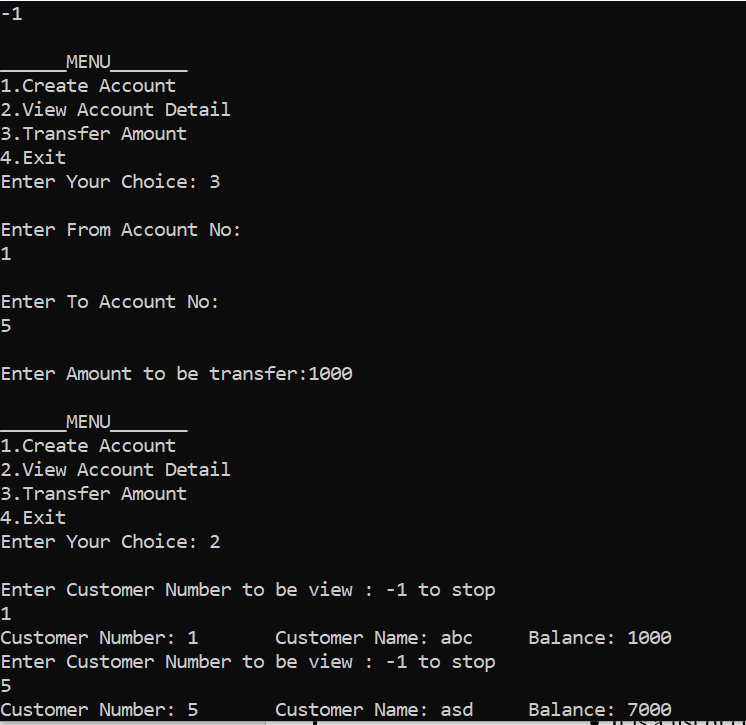
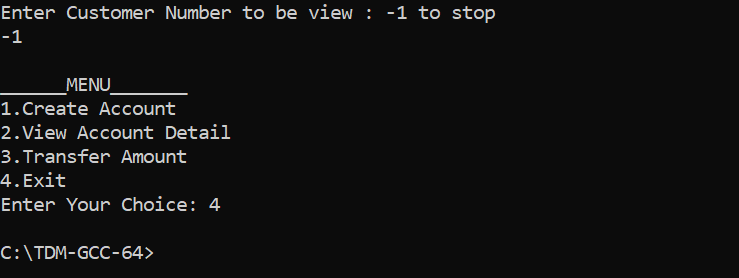
Views: 0