Table of Contents
show
- reads data from the given stream into the array pointed to, by ptr.
- Function fread transfers a specified number of bytes from the location in the file specified by the file position pointer to an area in memory beginning with a specified address. N
Syntax
fread
size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream)
Parameters
- ptr − This is the pointer to a block of memory with a minimum size of size*nmemb bytes.
- size − This is the size in bytes of each element to be read.
- nmemb − This is the number of elements, each one with a size of size bytes.
- stream − This is the pointer to a FILE object that specifies an input stream.
Return Value
The total number of elements successfully read are returned as a size_t object, which is an integral data type. If this number differs from the nmemb parameter, then either an error had occurred or the End Of File was reached.
Program: reading data from file using fread
#include <stdio.h>
#include<stdlib.h>
struct marks
{
int m1, m2,m3,m4,m5;
};
int main()
{
int n;
struct marks m;
FILE *fptr;
if ((fptr = fopen("mark.bin","rb")) == NULL){
printf("Cannot Open File !");
exit(1);
}
printf("Marks are\n");
for(n = 1; n <= 5; ++n)
{
fread(&m, sizeof(struct marks), 1, fptr);
printf("Student %d Marks : English: %d\t Maths : %d\t Physics: %d\t Chemistry : %d\t Python: %d\n",n, m.m1, m.m2, m.m3,m.m4,m.m5);
}
fclose(fptr);
return 0;
}
Output
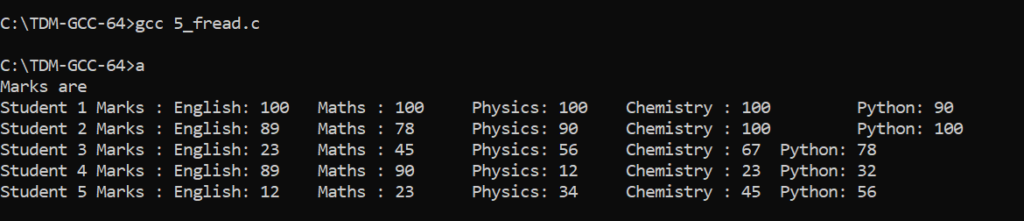
Views: 0