Table of Contents
show
The standard library provides many functions for reading data from files and for writing data to files
- Reads one character from a file.
- Function fgetc receives as an argument a FILE pointer for the file from which a character will be read.
- The call fgetc(stdin) reads one character from stdin—the standard input.
- This call is equivalent to the call getchar().
- After reading the character, the file pointer is advanced to next character.
- Â If pointer is at end of file or if an error occurs EOF file is returned by this function.
Syntax
fgetc
int fgetc(FILE *pointer)
This function returns the ASCII code of the character read by the function
Program
#include <stdio.h>
int main ()
{
// open the file
FILE *fp = fopen("test.txt","r");
// Return if could not open file
if (fp == NULL)
return 0;
do
{
// Taking input single character at a time
char c = fgetc(fp);
// Checking for end of file
if (feof(fp))
break ;
printf("%c", c);
} while(1);
fclose(fp);
return(0);
}
Input
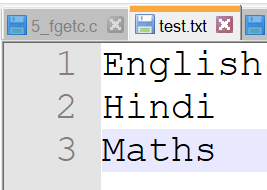
Output
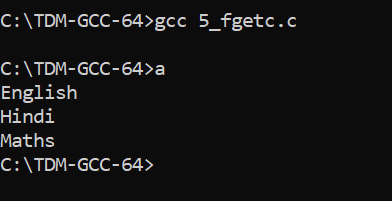
Views: 0