Table of Contents
show
- writes data from the array pointed to, by ptr to the given stream
- Function fwrite transfers a specified number of bytes beginning at a specified location in memory to a file.
- The data is written beginning at the location in the file indicated by the file position pointer
Syntax
fwrite
size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream)
Parameters
ptr − This is the pointer to the array of elements to be written.
size − This is the size in bytes of each element to be written.
nmemb − This is the number of elements, each one with a size of size bytes.
stream − This is the pointer to a FILE object that specifies an output stream.
Return Values
This function returns the total number of elements successfully returned as a size_t object, which is an integral data type. If this number differs from the nmemb parameter, it will show an error.
Program: writing data to file using fwrite
#include <stdio.h>
#include<stdlib.h>
struct marks
{
int m1, m2,m3,m4,m5;
};
int main()
{
int n;
struct marks m;
FILE *fptr;
if ((fptr = fopen("mark.bin","wb")) == NULL){
printf("File Cannot Open!");
exit(1);
}
printf("Enter 5 Students Marks\n");
for(n = 1; n <= 5; ++n)
{
printf("Enter English Mark of Student %d : ", n);
scanf("%d",&m.m1);
printf("Enter Maths Mark of Student %d : ", n);
scanf("%d",&m.m2);
printf("Enter Physics Mark of Student %d : ", n);
scanf("%d",&m.m3);
printf("Enter Chemistry Mark of Student %d : ", n);
scanf("%d",&m.m4);
printf("Enter Python Mark of Student %d : ", n);
scanf("%d",&m.m5);
fwrite(&m, sizeof(struct marks), 1, fptr);
}
fclose(fptr);
return 0;
}
Output
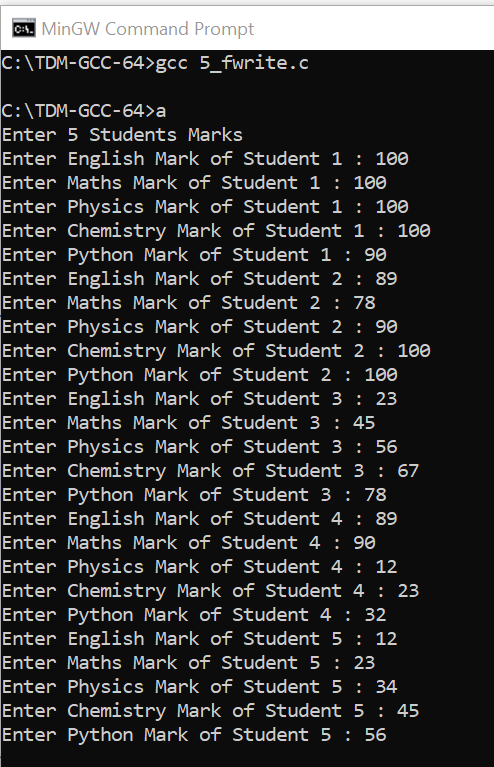
Views: 0